6 tips for ensuring fast Vue.js performance
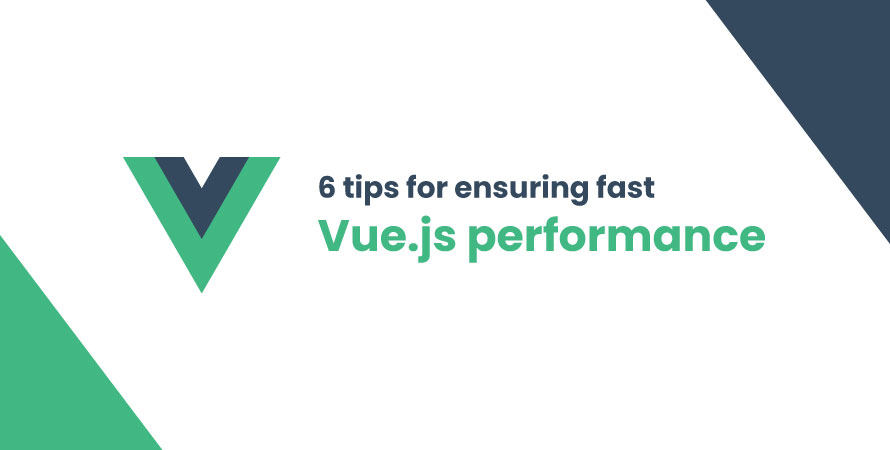
Vue.js has become one of the most popular JavaScript libraries in the web development community. It is loved for its simplicity and flexibility, providing developers with a lightweight yet powerful solution for creating user interfaces and single-page applications (SPAs). Its easy learning process and detailed documentation make it appealing to both newbies and seasoned developers.
However, even the strongest frameworks can face performance problems if not handled correctly. Dealing with these issues is crucial to deliver a smooth user experience, especially in critical areas like eCommerce. Performance problems in Vue.js projects can result in:
- Slower page loading times.
- Higher bounce rates.
- Lower search engine rankings.
- Decreased user satisfaction.
Optimizing Vue.js applications isn’t just a good idea; it’s necessary to stay ahead in today’s fast-paced digital world. The next sections will explore six practical tips to assist you in improving the performance of your Vue.js projects, making sure they operate smoothly and effectively.
Understanding the Key Factors Affecting Vue.js Performance
Importance of Vue.js Performance in eCommerce
Ensuring optimal performance in Vue apps is vital for delivering a seamless user experience, especially in eCommerce. Fast web application speed and load times are crucial as they directly influence customer satisfaction and conversion rates. Slow-loading pages can lead to higher bounce rates and abandoned shopping carts, which negatively affect sales and customer retention.
Impact of eCommerce Store Size and Complexity
The size and complexity of an eCommerce store can significantly impact Vue.js performance. Large stores with extensive product catalogs, numerous third-party integrations, and intricate user interfaces can create potential bottlenecks. These complexities can slow down page loads and degrade the overall site performance.
Key Factors:
- Product Catalog Size: Larger catalogs increase data handling needs.
- Third-Party Services: Integrations like payment gateways or search services add extra layers of processing.
- User Interface Complexity: Rich interactive elements require more rendering power.
Addressing these factors is essential for maintaining high-performance levels in Vue.js eCommerce applications.
Implementing Server-Side Rendering (SSR)
Server-Side Rendering (SSR) is a performance optimization technique where the rendering of web pages occurs on the server instead of the client-side browser. This method can significantly enhance initial page load speed by delivering pre-rendered HTML content to users, allowing them to interact with the page faster without waiting for JavaScript to fully load and execute.
What is SSR and How It Improves Performance?
Server-Side Rendering (SSR) is an application optimization technique where the rendering of web pages occurs on the server instead of the client-side browser. This method can significantly enhance initial page load speed by delivering pre-rendered HTML content to users, allowing them to interact with the page faster without waiting for JavaScript to fully load and execute.
Key Benefits of SSR:
- Faster Initial Load Times: By rendering HTML on the server, users receive a fully-formed page quickly, leading to a better user experience.
- Improved Search Engine Optimization (SEO): Since search engines can crawl pre-rendered HTML more efficiently than JavaScript-heavy content, SSR can enhance a website’s visibility in search engine results.
Benefits of SSR in Vue.js eCommerce Applications
In the context of Vue applications, implementing SSR can provide several advantages:
- Enhanced SEO-Friendliness: For eCommerce sites, appearing high in search engine results is crucial. SSR ensures that web crawlers from search engines like Google can easily index your site’s content, improving its ranking.
- Reduced Client-Side Rendering Load: With SSR, most of the heavy lifting is done on the server. This reduces the reliance on client-side JavaScript for rendering pages, which is particularly beneficial for users with slower internet connections or less powerful devices. it is very helpful in optimizing applications.
Optimizing Data Handling with Vuex State Management
Vuex is a state management library designed to work seamlessly with Vue.js applications. It centralizes the application’s state into a single store, enabling predictable state mutations and providing a structured development pattern. Effective state management is crucial for performance optimization in Vue.js eCommerce projects.
Overview of Vuex and Its Role
Vuex operates on four core concepts:
- State: Represents the single source of truth for application data.
- Getters: Allow you to compute derived state based on store state.
- Mutations: Synchronous operations that directly modify the state.
- Actions: Asynchronous operations that can commit mutations.
By centralizing state management, Vuex reduces the complexity of handling shared state between components, leading to more maintainable and scalable applications.
Best Practices for Efficient Data Handling
Efficiently using Vuex involves organizing modules and distinguishing between mutations and actions properly:
Module Organization
Large applications benefit from breaking down the Vuex store into smaller, manageable modules. Each module can contain its own state, getters, mutations, and actions. This modular approach enhances code readability and maintainability.
javascript const userModule = { state: () => ({ userInfo: null, }), getters: { getUserInfo(state) { return state.userInfo; }, }, mutations: { setUserInfo(state, payload) { state.userInfo = payload; }, }, actions: { fetchUserInfo({ commit }) { // Fetch user info from an API commit(‘setUserInfo’, fetchedData); }, }, };
Proper Use of Mutations vs Actions
- Mutations should always be synchronous and are responsible for changing the state directly.
- Actions, on the other hand, handle asynchronous tasks like API calls before committing mutations to update the state.
Using this separation helps maintain a clear flow of data within the application and prevents unwanted side effects from asynchronous operations.
Caching API Responses with Axios and Redis
The Concept of Caching in Vue.js eCommerce Projects
Caching is a technique that stores copies of files or data in a cache, or temporary storage location, to reduce the time it takes to access this data and decrease the number of server round-trips. For Vue.js eCommerce projects, caching significantly enhances performance by ensuring that frequently requested data is readily available without repeatedly querying the server.
Implementing API Response Caching Strategies
To effectively implement API response caching in Vue.js applications, two popular tools come into play: Axios for making HTTP requests and Redis as a caching server.
Using Axios for HTTP Requests
Axios is a promise-based HTTP client for JavaScript, widely used for making requests to APIs. It allows developers to set up interceptors that can handle responses before they are processed by the application. An example implementation:
javascript import axios from ‘axios’;
// Create an Axios instance const apiClient = axios.create({ baseURL: ‘https://api.example.com’, timeout: 1000, });
// Set up request interceptor to cache responses apiClient.interceptors.response.use(response => { // Assume we have a cache object to store responses const cachedResponse = cache.get(response.config.url); if (cachedResponse) { return Promise.resolve(cachedResponse); }
// Store the response in cache cache.set(response.config.url, response.data);
return response; }, error => { return Promise.reject(error); });
export default apiClient;
Using Component-Level Lazy Loading
When it comes to optimizing app performance of Vue.js, one technique stands out: lazy loading. By loading components only when they are needed, lazy loading helps reduce the initial bundle size and delays the execution of non-essential code.
Benefits of Lazy Loading
- Reduced Initial Bundle Size: By loading only the necessary components at the beginning, we can minimize the amount of JavaScript that needs to be downloaded and parsed initially.
- Delayed Loading of Non-Essential Code: Components that are not crucial to the initial page view are loaded only when required, which means they don’t contribute to the initial load times, thus enhancing the user experience.
- Improved App Performance: By splitting code into smaller chunks and loading them on demand, lazy loading ensures smoother navigation and interaction within the application.
Implementing Lazy Loading with Vue Router
Vue Router makes it simple to implement lazy loading through dynamic imports. Here’s how you can do it:
Install Vue Router:
bash npm install vue-router
Configure Routes with Dynamic Imports:
javascript import Vue from ‘vue’; import Router from ‘vue-router’;
Vue.use(Router);
const routes = [ { path: ‘/home’, component: () => import(/* webpackChunkName: “home” /
‘./components/Home.vue’) }, { path: ‘/about’, component: () => import(/ webpackChunkName: “about” */
‘./components/About.vue’) } ];
const router = new Router({ mode: ‘history’, routes });
export default router;
In this configuration:
The component property uses a dynamic import statement (import()). This ensures that each component is loaded only when its route is accessed.
The webpackChunkName comment helps in naming the chunks for easier debugging and caching.
Fine-Tuning Virtual DOM Performance
Understanding the Virtual DOM is essential for achieving optimal performance in Vue.js applications. The Virtual DOM is a lightweight representation of the actual DOM, allowing Vue.js to update only the parts of the DOM that have changed, rather than re-rendering the entire page. This selective updating mechanism significantly enhances rendering performance and responsiveness.
Concept of the Virtual DOM in Vue.js
In Vue.js, when the state of an application changes, a new Virtual DOM tree is created. Vue then compares this new tree with the previous one using a process called diffing. Once it identifies differences between the two trees, Vue applies these changes to the real DOM in an efficient manner.
Benefits of using the Virtual DOM include:
- Improved Rendering Speed: By updating only what has changed, rendering is faster and less resource-intensive.
- Easier State Management: Changes in application state are more predictable and manageable.
Best Practices for Optimizing Virtual DOM Updates
To fully leverage the benefits of the Virtual DOM, developers need to follow best practices for optimizing updates:
Use the key Attribute Correctly:
- The key attribute helps Vue identify which items have changed, been added, or removed from lists. Each key should be unique among its siblings.
- Example:
- html
- Proper usage of key ensures efficient re-rendering by reducing unnecessary updates.
Minimize Re-renders:
- Avoid making unnecessary state changes that trigger re-renders. Only update state when absolutely necessary.
- Example:
- javascript // Inefficient this.items.push(newItem);
- // Efficient this.$set(this.items, index, newItem);
Optimize Component Hierarchy:
- Break down complex components into smaller ones to isolate re-renders.
- Example:
- html
Use Functional Components:
- For components that do not need their own state or lifecycle methods, use functional components to reduce overhead.
- Example:
- javascript Vue.component(‘functional-component’, { functional: true, render (createElement, context) { return createElement(‘div’, context.props.message); } });
Adopting these practices can lead to significant improvements in application performance by ensuring efficient updates and minimizing unnecessary re-renders. This makes your Vue.js applications more responsive and performant, directly enhancing user experience.
Measuring and Analyzing Vue.js App Performance
Ensuring optimisation of app performance in a Vue.js application requires continuous measurement and monitoring. Performance profiling helps identify bottlenecks and areas that need improvement, ultimately leading to a faster and more responsive user experience.
Importance of Ongoing Performance Measurement
Regular performance analysis is crucial for maintaining an efficient Vue.js application. As the app evolves, new features and changes can introduce performance issues. Identifying these problems early allows developers to address them before they impact the user experience.
Key aspects include:
- Tracking load times to ensure swift navigation.
- Monitoring resource usage to prevent unnecessary strain on devices.
- Analyzing user interactions to detect any sluggish behavior.
Tools for Performance Profiling
Several tools can help developers measure and analyze the performance of their Vue.js applications effectively:
Vue Devtools
Vue Devtools is an essential extension for debugging and profiling Vue.js applications. It offers several features to help developers understand their app’s performance:
- Component Inspector: Allows you to inspect components’ data, props, events, and lifecycle hooks.
- Performance Tab: Provides a timeline of component updates, helping you spot inefficient renders or excessive reactivity.
- State Management: Visualizes your Vuex state tree, making it easier to track state changes and identify potential issues.
Conclusion
Applying these six tips can greatly enhance the performance of your Vue.js applications. Server-Side Rendering (SSR) improves load speed and search engine visibility, while optimizing data handling with Vuex ensures efficient state management. Caching API responses with Axios and Redis speeds up data retrieval, and component-level lazy loading reduces the initial bundle size.
Fine-tuning the Virtual DOM boosts rendering speed, and regular performance monitoring ensures sustained optimization. Balancing performance with usability and maintainability creates fast, efficient, and user-friendly applications, ensuring higher engagement and satisfaction.