Step-by-Step Guide To Create Microservices With Node.js
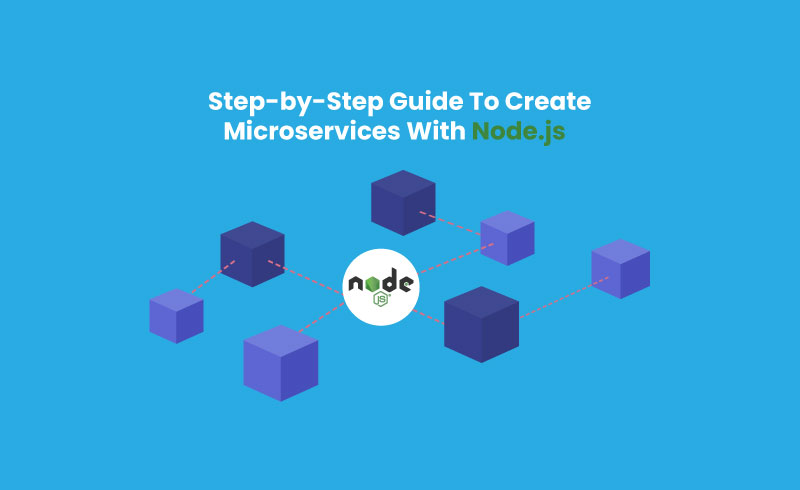
Microservices development represent a new way of developing software applications, where large applications are broken down into smaller, interconnected services. This service-oriented architecture (SOA) improves flexibility, scalability, reliability, and maintainability.
Nodejs microservice is a powerful, open-source JavaScript runtime environment used for server-side and networking applications. It works on different operating systems like OS X, Linux, and Microsoft Windows. Node.js is known for its speed in executing code with the V8 JavaScript Engine and its ability to handle multiple requests efficiently using an event loop system.
Importance of Microservices in Modern Software Development
- Scalability: Each Node JS microservice can be scaled independently based on demand.
- Flexibility: Different microservices in node can be built using different programming languages or technologies.
- Resilience: If one Node microservice fails, it doesn’t affect the entire system.
- Maintainability: It’s easier to update or deploy small parts of the application without impacting the whole system.
Benefits of Using Node.js for Building Microservices
- High Performance: The V8 engine allows for faster execution of code.
- Efficient Handling of Multiple Requests: Uses an event-driven, non-blocking input/output model.
- Real-Time Applications: Enables the creation of high-performance real-time web applications.
- Community and Ecosystem: A wide range of packages available through npm (Node Package Manager).
Companies like Yammer, Uber, and Microsoft use Node.js extensively to develop efficient microservices architectures.
Prerequisites
Before we begin, make sure you have Node.js installed on your machine. Node js architecture is an open-source JavaScript runtime environment that allows you to run server-side code.
To check if Node.js is installed, open your terminal and run the following command:
bash node -v
You should see a version number displayed. If Node.js is not installed, you can download and install it from the official website.
This microservices tutorial assumes you are using at least version 8.11 of Node.js.
Determining Business Needs
Understanding the specific requirements that will shape your microservices architecture NodeJS is crucial. Business needs to drive the design and functionality of each microservice, ensuring that they align with overall business goals. Here are some key aspects to consider:
- Identify Core Services: Break down your application into core functionalities that can be turned into independent services.
- Scalability Requirements: Determine which microservices in Node JS need to scale independently based on varying demands.
- Data Management: Assess how data will be managed and shared across different microservices.
- Performance Metrics: Define performance expectations for each service to ensure they meet user needs.
- Security Needs: Understand the security requirements to protect sensitive data and maintain compliance.
Taking these factors into account helps create a solid foundation for your microservices in NodeJS architecture, tailored to your specific business requirements.
Setting up the Node.js Project
To begin, you’ll need to build with the Node js project. Open your terminal and navigate to your project directory. Run the following command:
bash npm init -y
This command will create a package.json file, which serves as the blueprint for your Node.js project, storing metadata and dependencies. Next, install essential packages like Express for handling HTTP requests:
bash npm install express
Additionally, consider installing tools like Nodemon for automatic server restarts during development:
bash npm install –save-dev nodemon
Now, create an entry point file named server.js where you will configure your Express server.
Building Individual Microservices
Defining Service Contracts with RESTful APIs
You need to learn how to build a microservices architecture with Node.js. When building Nodejs microservices, defining service contracts using RESTful APIs is an essential step. the Express JS RESTful APIs facilitate clear communication between different microservices, ensuring that each service can interact seamlessly with others.
Key Components of a Service Contract
- Endpoints: Define the paths through which clients can access resources.
- HTTP Methods: Specify the type of action to be performed, such as GET, POST, PUT, DELETE.
- Request Parameters: Include path parameters, query parameters, and request bodies necessary for the API to process the request.
- Response Format: Standardize the structure of responses (usually in JSON format) to ensure consistency.
Example API Contract
json { “endpoint”: “/api/v1/users”, “methods”: { “GET”: { “description”: “Retrieve a list of users”, “parameters”: { “query”: { “type”: “string”, “required”: false, “description”: “Filter users by type” } }, “responses”: { “200”: { “description”: “A list of users”, “content”: { “application/json“: { “schema”: { “$ref”: “#/components/schemas/UserList” } } } }, “400”: { “description”: “Invalid request” } } }, … } }
Benefits of Well-Defined API Contracts
- Clarity: Reduces ambiguity in how nestjs microservices should interact.
- Interoperability: Ensures that microservices built by different teams or technologies can work together.
- Scalability: Facilitates scaling individual components without breaking the overall system.
Defining these contracts before implementation helps create a robust foundation for your microservices architecture.
Implementing Data Storage for Microservices
Data persistence is crucial when building microservices. Each microservice may have its own database to ensure loose coupling and independence.
Approaches to Data Persistence:
- Relational Databases: Suitable for structured data and complex queries.
- NoSQL Databases: Ideal for unstructured data, offering flexibility and scalability.
- In-Memory Databases: Great for caching and real-time data access.
Nodejs Example: Using MongoDB with Node.js:
javascript const mongoose = require(‘mongoose’);
mongoose.connect(‘mongodb://localhost:27017/mydatabase’, { useNewUrlParser: true, useUnifiedTopology: true }); const db = mongoose.connection;
db.on(‘error’, console.error.bind(console, ‘connection error:’)); db.once(‘open’, function() { console.log(“We’re connected!”); });
This code snippet establishes a connection to a MongoDB instance, providing a foundation for robust data storage in your microservices architecture.
Implementing Business Logic in Microservices
Building microservices involves encapsulating the unique functionality of each service. In this step, we will dive into the core implementation of each microservice, following a consistent pattern:
Define the Business Logic
Identify the specific tasks each microservice needs to perform.
Use controllers to handle incoming requests and direct them to appropriate service functions.
Implement Controller Functions
Write handler functions that encapsulate your business logic.
For example, if you have a UserService, you might implement functions like createUser, getUserById, and updateUser.
Use Models for Data Manipulation
Interact with the data layer using models.
Apply necessary transformations and validations before processing data.
javascript // Example: UserController.js const UserModel = require(‘../models/UserModel’);
exports.createUser = async (req, res) => { try { const user = await UserModel.create(req.body); res.status(201).json(user); } catch (error) { res.status(500).json({ message: error.message }); } };
Each microservice should be self-contained, with its business logic clearly defined and modularized. This ensures maintainability and scalability across your application.
Ensuring Resilience and Fault Tolerance
Building microservices with resilience and fault tolerance is critical for maintaining a robust system. In this step, we will dive into the core implementation of each microservice, following a consistent pattern:
Circuit Breakers
Use libraries like opossum to implement circuit breakers, preventing cascading failures by stopping calls to a failing service.
Retries and Timeouts
Configure retries with exponential backoff strategies and set appropriate timeouts to handle transient failures effectively.
Fallback Mechanisms
Design fallback responses or services to provide partial functionality when a dependency fails.
Health Checks
Implement regular health checks using tools like node-health-check to monitor the status of each microservice.
Bulkheads
Isolate resources such as thread pools or connection pools to prevent failure in one part of the system from affecting others.
These techniques help ensure your microservices can manage failures gracefully without impacting overall system performance.
Orchestrating Microservices Communication
Effective microservices communication is crucial for a well-functioning architecture. Managing interactions between different microservices involves:
- API Gateway: Acts as a single entry point for all client requests, routing them to appropriate microservices.
- Service Discovery: Dynamically finds the network locations of microservice instances.
- Message Brokers: Facilitates asynchronous communication between services using message queues or topics.
- Circuit Breakers: Prevents cascading failures by isolating failing services.
By implementing these strategies, you ensure seamless and efficient interaction among your microservices.
Securing Microservices
Implementing robust security measures for your microservices is crucial to protect them from unauthorized access and malicious attacks. Consider the following strategies:
- Authentication and Authorization: Use strong authentication mechanisms such as OAuth2 or JWT to verify the identity of users and services. Implement role-based access control (RBAC) to ensure that only authorized entities can access specific resources.
- Encryption: Protect data in transit by using HTTPS with TLS encryption. Encrypt sensitive data at rest using industry-standard algorithms.
- API Gateway: Utilize an API Gateway to centralize and manage security policies, rate limiting, and request validation across all microservices.
- Regular Audits and Monitoring: Conduct regular security audits and implement monitoring tools to detect and respond to suspicious activities promptly.
You can safeguard your daily code buffer microservices architecture against potential threats by following these practices.
Testing and Debugging Microservices
Ensuring the quality and reliability of your microservices requires a robust approach to testing and debugging.
Strategies for Testing Microservices
- Unit Testing: Verify individual components of each microservice.
- Integration Testing: Ensure communication between microservices operates as expected.
- End-to-End Testing: Test the entire workflow from start to finish to uncover any issues in the overall architecture.
- Contract Testing: Validate that service contracts (APIs) between services are adhered to, ensuring reliable interactions.
Strategies for Debugging Microservices
- Logging: Implement comprehensive logging to trace issues effectively.
- Monitoring Tools: Use tools like Prometheus or ELK Stack for real-time monitoring and alerting.
- Distributed Tracing: Utilize tools such as Jaeger or Zipkin to trace requests across multiple services, pinpointing where failures occur.
Applying these strategies fosters a resilient, high-quality microservices architecture capable of handling real-world demands.
Deploying Microservices
Deploying microservices to a production environment involves several options:
- Containerization: Using Docker to package your Node.js microservices into containers, ensuring consistency across different environments.
- Orchestration: Employing Kubernetes for managing and scaling your containerized applications.
- Cloud Services: Utilizing platforms like AWS, Google Cloud Platform, or Microsoft Azure for deploying and managing microservices.
- Serverless Architectures: Leveraging services such as AWS Lambda or Azure Functions to deploy functions as a service, reducing the need for managing infrastructure.
Ensuring proper deployment strategies guarantees the scalability and reliability of your microservices in production.
Conclusion
Creating microservices with Node.js offers a powerful solution for modern software development by breaking down monolithic applications into smaller, independent services, enhancing both modularity and scalability. Node.js is an ideal choice for this architecture due to its high performance, driven by the V8 JavaScript Engine, its non-blocking I/O that handles multiple requests simultaneously, and its real-time capabilities, making it perfect for high-performance web applications. When you hire a Node.js developer, they can guide you through the essential steps, from identifying business needs to deploying services, to build robust, scalable, and maintainable microservices. Ensuring clear API contracts, solid data storage strategies, fault tolerance, and strong security measures is crucial for creating reliable and secure services. Testing and debugging are also vital stages, helping to catch issues early and ensure functionality. Finally, deploying your microservices in a production environment prepares them for real-world use. Hiring a skilled Node.js developer will not only help you leverage the advantages of Node.js but also ensure your microservices architecture aligns with the demands of modern software development. This guide provides a roadmap to navigate the process efficiently.