Unit Testing in Flutter: A Comprehensive Guide
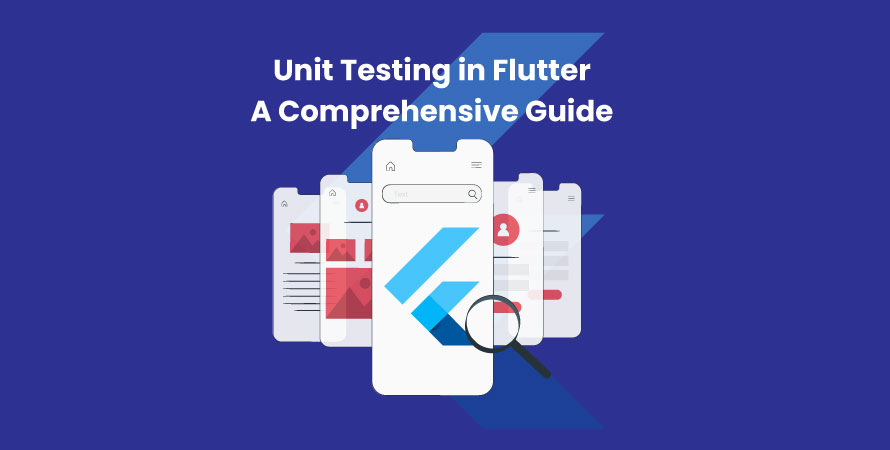
Unit testing in Flutter is an essential practice that ensures individual components of an application function correctly. By isolating and verifying specific sections of code, unit tests help maintain the robustness and reliability of Flutter applications. The importance of unit testing for Android cannot be overstated, as it allows developers to catch bugs early in the development process, leading to more stable and high-performance applications.
Key Takeaway: Effective unit testing is crucial for building reliable and high-performance Flutter applications.
In this guide, you’ll learn:
- The fundamentals of unity test setup in software development.
- How to set up a unit testing environment in your Flutter project.
- Techniques for writing effective and maintainable unit tests.
- Common Flutter code examples of unit testing scenarios in projects.
- Best practices to enhance your API unit testing strategy.
- Advanced features and techniques are available within Flutter’s testing frameworks.
- Methods for running your unit tests efficiently.
By the end of this guide, you’ll have a comprehensive understanding of how to implement and leverage unit testing to ensure the quality and reliability of your Flutter applications.
Understanding Unit Testing
Definition and Purpose of Unit Testing
Unit testing is a fundamental practice in software development where individual units or components of the software are tested in isolation. A unit typically refers to the smallest part of an application, such as a function or method. The primary purpose of unit testing is to verify that each unit performs as expected and adheres to its specified behavior.
Importance of Verifying Isolated Sections of Code
Verifying isolated sections of code ensures that your application is robust and maintainable. By focusing on small and specific parts of your codebase, you can:
- Catch Bugs Early: Identify issues at an early stage in the development process, which simplifies debugging and reduces the cost of fixing defects.
- Ensure Reliability: Confirm that each component works correctly under various scenarios, leading to a more reliable overall application.
- Facilitate Refactoring: Make changes to the code with confidence, knowing that existing functionality remains intact through comprehensive tests.
- Enhance Documentation: Serve as living documentation for the codebase, making it easier for new developers to understand the intended behavior.
Differences Between Unit Tests and Other Types of Tests
It’s important to distinguish between unit tests and other types of tests to understand their unique roles:
- Unit TestsScope: Test individual functions or methods in isolation.
- Focus: Validate logic correctness within a single unit.
- Speed: Typically very fast due to their limited scope.
- Integration TestsScope: Test how multiple components work together.
- Focus: Ensure different parts of the application interact correctly.
- Complexity: Involves setting up environments and if else Flutter dependencies.
- Widget Tests (in Flutter)Scope: Test individual widgets and their interactions within the UI.
- Focus: Verify visual elements and user interactions.
- Balance: Combine aspects of both unit tests (isolated logic) and Flutter test assets (component interactions).
Understanding these differences helps you choose the appropriate test type for various aspects of your application, ensuring comprehensive test coverage while maintaining efficiency.
Setting Up the Unit Testing Environment
Adding the flutter_test Package
To start unit testing in a Flutter define project, you need to add the flutter_test package as a development dependency. Follow these steps:
- Open your pubspec.yaml file.
- Under the dev_dependencies section, add the flutter_test package:
- yaml dev_dependencies: flutter_test: sdk: flutter
- Run flutter pub get to fetch the new dependencies.
This setup allows you to leverage Flutter’s robust testing framework to write and execute unit tests.
Organizing Test Files
A well-structured directory makes managing and scaling your tests easier. Here’s a recommended directory structure for organizing unit tests in Flutter projects:
- Project Rootlib/: Contains your application’s source code.
- test/: Houses all test files.
Within the test/ directory, mirror the structure of your lib/ directory. This approach helps keep your tests organized and easy to locate.
Example Directory Structure
Suppose your project has the following structure in the lib/ folder:
lib/ ├── models/ │ └── counter.dart └── services/ └── database_service.dart
Your test/ directory should mirror this structure:
test/ ├── models/ │ └── counter_test.dart └── services/ └── database_service_test.dart
Naming Conventions
Use descriptive names for your test files by appending _test.dart to the corresponding source file name. For instance, the test file for counter.dart should be named counter_test.dart. This dart testing convention simplifies identifying which tests correspond to which parts of your application.
Example of Adding a Test File
Here’s how you might set up a basi testing for a simple counter class in the test/models/counter_test.dart file:
dart import ‘package:flutter_test/flutter_test.dart’; import ‘package:your_project/models/counter.dart’;
void main() { group(‘Counter’, () { test(‘should increment value’, () { final counter = Counter();
counter.increment();
expect(counter.value, 1);
});
test(‘should decrement value’, () {
final counter = Counter();
counter.decrement();
expect(counter.value, -1);
});
}); }
These Flutter examples demonstrate how to organize and implement basic unit tests in Flutter effectively.
By structuring your project files logically and adding necessary dependencies, setting up an efficient unit testing environment becomes manageable.
Writing Effective Unit Tests in Flutter
Effective unit testing in Flutter relies on understanding the basic structure of a test and employing best practices to ensure clarity and maintainability. This section will guide you through using the test and expect functions, exploring the arrange-act-assert pattern, and leveraging mocking with the Mockito library.
Breakdown of a Typical Unit Test Structure
Unit tests in Flutter integration testing are written using the test function, which defines a single test case. The expect function is then used to assert the expected outcomes. Here’s a basic example:
dart import ‘package:flutter_test/flutter_test.dart’;
void main() { test(‘Simple addition test’, () { // Arrange int result;
// Act
result = 2 + 3;
// Assert
expect(result, 5);
}); }
In this example:
- Arrange: Set up any variables or state needed.
- Act: Perform the action to be tested.
- Assert: Verify that the action results in the expected outcome.
Arrange-Act-Assert Pattern
The arrange-act-assert pattern is fundamental for writing clear and maintainable tests:
- Arrange: Initialize objects, set up mock data, or configure any prerequisites.
- Act: Execute the method or function being tested.
- Assert: Check that the output matches expectations.
This pattern helps in keeping tests structured and easy to read. For example:
dart class Counter { int value = 0;
void increment() => value++; }
void main() { test(‘Counter increments value’, () { // Arrange final counter = Counter();
// Act
counter.increment();
// Assert
expect(counter.value, 1);
}); }
Understanding Mocking Dependencies with Mockito
In real-world applications, it’s often necessary to isolate dependencies to test specific parts of your codebase effectively. This is where mocking comes into play. The Mockito library is commonly used for this purpose in Flutter.
To use Mockito:
- Add mockito as a dev dependency in your pubspec.yaml: yaml dev_dependencies:mockito: ^5.0.0
- Create a mock class using Mockito annotations: dart import ‘package:mockito/annotations.dart’; import ‘package:mockito/mockito.dart’;
- class Dependency { String fetchData() => ‘Real Data’; }
- @GenerateMocks([Dependency]) void main() {}
- Use the mock class in your tests:
dart import ‘package:flutter_test/flutter_test.dart’; import ‘package:mockito/annotations.dart’; import ‘package:mockito/mockito.dart’; import ‘path/to/generated_file.mocks.dart’; // Import generated mock file
@GenerateMocks([Dependency]) void main() { late MockDependency mockDependency;
setUp(() { mockDependency = MockDependency(); });
test(‘Fetch data returns mocked data’, () { // Arrange when(mockDependency.fetchData()).thenReturn(‘Mocked Data’);
// Act
final result = mockDependency.fetchData();
// Assert
expect(result, ‘Mocked Data’);
}); }
Using Mockito allows you to control and predict dependencies’ behavior, making your unit tests more robust and focused on specific functionalities without interference from external factors.
Understanding these concepts ensures your unit tests are effective, isolated, and reliable, paving the way for maintaining high-quality Flutter applications with ease.
Common Examples of Unit Testing in Flutter Projects
Testing a Simple Counter Class
A common scenario in Flutter is testing a simple counter class. This example covers different scenarios like incrementing and decrementing values. Below is an overview of how to write unit tests for such a class.
Counter Class Definition:
dart class Counter { int value = 0;
void increment() => value++; void decrement() => value–; }
Unit Test for Counter Class:
- Create the test file: Create a file named counter_test.dart in the dart test directory.
- Write test cases: Use the test function to define each test case and expect to assert the outcomes.
dart import ‘package:flutter_test/flutter_test.dart’; import ‘path/to/counter.dart’; // Update with correct path
void main() { group(‘Counter’, () { test(‘initial value should be 0’, () { final counter = Counter(); expect(counter.value, 0); });
test(‘value should increment by 1’, () {
final counter = Counter();
counter.increment();
expect(counter.value, 1);
});
test(‘value should decrement by 1’, () {
final counter = Counter();
counter.decrement();
expect(counter.value, -1);
});
}); }
This setup verifies that the Counter class behaves as expected when its methods are called.
Testing CRUD Operations within a Database Class
Testing database operations ensures that your app’s data management logic works correctly. Here’s an example demonstrating how to test CRUD operations using mock data and assertions.
Database Class Definition (Using a Mock):
Assume we have a DatabaseService class with methods for Create, Read, Update, and Delete (CRUD) operations.
dart class DatabaseService { List _items = [];
void createItem(String item) => _items.add(item);
String readItem(int index) => _items[index];
void updateItem(int index, String newItem) => _items[index] = newItem;
void deleteItem(int index) => _items.removeAt(index); }
Unit Test for Database Class:
- Add Mockito dependency: Add the mockito package in your pubspec.yaml.
yaml dev_dependencies: flutter_test: sdk: flutter mockito: ^5.0.0 # Check for the latest version
- Create the mock class: Extend the Mock class from Mockito.
dart import ‘package:mockito/mockito.dart’;
class MockDatabaseService extends Mock implements DatabaseService {}
- Write test cases: Use Mockito to verify method calls and outcomes.
dart import ‘package:flutter_test/flutter_test.dart’; import ‘package:mockito/mockito.dart’; import ‘path/to/database_service.dart’; // Update with correct path
void main() { group(‘DatabaseService’, () { final db = MockDatabaseService();
setUp(() {
// Optional: reset or initialize state before each test
});
test(‘should add an item’, () {
db.createItem(‘item1’);
verify(db.createItem(‘item1’)).called(1);
expect(db.readItem(0), ‘item1’);
});
test(‘should read an item’, () {
when(db.readItem(0)).thenReturn(‘item1’);
var item = db.readItem(0);
expect(item, ‘item1’);
});
test(‘should update an item’, () {
db.createItem(‘old_item’);
db.updateItem(0, ‘new_item’);
verify(db.updateItem(0, ‘new_item’)).called(1);
expect(db.readItem(0), ‘new_item’);
});
test(‘should delete an item’, () {
db.createItem(‘item_to_delete’);
db.deleteItem(0);
verify(db.deleteItem(0)).called(1);
});
}); }
Using these examples, you can see how unit tests help ensure that both simple and complex functionalities perform as expected in isolation.
Best Practices for Writing Unit Tests in Flutter Applications
Integrating Continuous Integration (CI) Practices
Continuous Integration (CI) is a crucial practice for automating test execution in response to code changes. By integrating CI into your development workflow, you ensure that unit tests are run automatically whenever there’s a new commit or pull request. This practice helps catch bugs early and maintains code quality consistently.
Popular CI Tools
- CircleCI: Offers robust support for Flutter projects, allowing seamless integration with GitHub and Bitbucket.
- Travis CI: Known for its simplicity and ease of use, Travis CI can be configured to run Flutter tests efficiently.
- GitHub Actions: Provides native support within GitHub repositories, enabling easy setup for automated testing workflows.
Monitoring Code Coverage
Code coverage monitoring is essential to ensure that all critical parts of your codebase are adequately tested. Code coverage tools provide insights into which lines of code are executed during tests, helping identify untested areas.
Tools for Code Coverage
- coverage package: A Dart package that generates detailed reports on test coverage.
- Codecov: Integrates with CI tools to visualize code coverage metrics and track changes over time.
Strategies for Effective Code Coverage Monitoring
Regularly Review Coverage Reports:
- Use generated reports to identify untested code sections.
- Prioritize writing tests for high-risk areas like core functionalities and business logic.
Set Coverage Thresholds:
- Define minimum acceptable coverage percentages (e.g., 80%) within your CI configuration.
- Ensure builds fail if the coverage drops below the threshold, maintaining a high standard of code quality.
Focus on Meaningful Tests:
- Aim to write tests that cover various scenarios, including edge cases and error conditions.
- Avoid writing superficial tests just to increase numbers; focus on meaningful assertions that verify the behavior of your application components.
Refactor Regularly:
- As the codebase evolves, regularly refactor both production and test code to maintain clarity and effectiveness.
- Ensure that tests remain up-to-date with any changes in the application’s functionality.
By integrating these best practices into your Flutter development workflow, you enhance the reliability and maintainability of your application through effective unit testing strategies. Automated CI processes combined with diligent code coverage monitoring create a robust testing environment conducive to high-quality software development.
Exploring Advanced Features and Techniques in Flutter Testing Frameworks
Golden File Testing
Golden file testing is a powerful technique for validating the visual appearance of UI components across different devices or screen sizes. This approach involves rendering your widget and capturing its visual output as a “golden” image. Subsequent test runs compare the current rendering with this golden image to detect any unintended changes.
Steps to Implement Golden File Testing:
- Set Up the Test Environment: Add the flutter_test package to your dev_dependencies in pubspec.yaml.
- Write the Golden Test: Create a test file that includes the widget you want to test and specify the golden file for comparison.
- Generate and Update Golden Files: Run the test to generate or update the golden file if necessary using:
- sh flutter test –update-goldens
Unit Tests vs Integration Tests
Understanding the distinctions between unit tests and integration tests is key to maintaining overall app stability.
Unit Tests:
- Focus: Verify isolated sections of code, typically functions or methods.
- Scope: Narrow, concentrating on individual units of functionality.
- Dependencies: Often use mocking to isolate external dependencies.
Example: Testing a counter class’s increment function.
Integration Tests:
- Focus: Ensure comprehensive functionality by testing multiple components working together.
- Scope: Broader, covering interactions between several parts of the application.
- Dependencies: Involve real or mocked services and databases.
Example: Testing user login flow from entering credentials to navigating after a successful login.
Using both types of tests allows you to address different aspects of your app’s functionality:
Unit tests help catch bugs early during development by verifying individual pieces of code.
Integration tests ensure that components work together correctly, providing confidence that your app functions as expected end-to-end.
Running Your Unit Tests Effectively in Flutter Projects
Running unit tests efficiently is crucial for maintaining a smooth development workflow in Flutter projects. Leveraging popular IDEs like IntelliJ IDEA and Visual Studio Code (VSCode) can significantly streamline this process.
Running Tests from IntelliJ IDEA
IntelliJ IDEA offers robust support for Flutter, making it easy to run unit tests directly from the IDE:
- Open the project: Ensure your Flutter project is open in IntelliJ IDEA.
- Navigate to the test file: Locate the test file you wish to run.
- Run the test: Right-click on the test file or specific test function.
- Select Run ‘testName‘ from the context menu.
- View results: The results will be displayed in the Run window, providing detailed information about passed and failed tests.
Running Tests from Visual Studio Code (VSCode)
VSCode also provides excellent support for running Flutter unit tests:
- Open the project: Open your Flutter project in VSCode.
- Install necessary extensions: Install the Flutter and Dart extensions from the VSCode marketplace if not already installed.
- Navigate to the test file: Open the test file you wish to execute.
- Run the test: Click on the green play icon next to each test function or at the top of the file.
- View results: Test outcomes will be shown in the OUTPUT panel or Terminal, indicating success or failure.
Command Line Execution
For those who prefer command line operations, flutter test offers a straightforward way to run all unit tests in your project:
- Open terminal: Navigate to your project’s root directory.
- Execute tests: bash flutter test
- Review results: The terminal will display a summary of test results, including any errors or failures.
Utilizing these methods ensures you can efficiently run and review your unit tests, helping maintain code quality across your Flutter applications.
Conclusion
Understanding the importance of effective unit testing is crucial for building robust applications. By adopting best practices like continuous integration (CI) to automate tests and monitor code coverage, developers can ensure consistent code quality. Regularly refactoring and updating tests keeps them relevant as your codebase evolves, making it easier to catch bugs early and maintain a healthy application.
Partner with Build Offshore Team for expert developers who can enhance your testing processes, ensuring your Flutter apps remain fast, maintainable, and bug-free throughout the development lifecycle.