The Ultimate Guide to Software Application Architecture
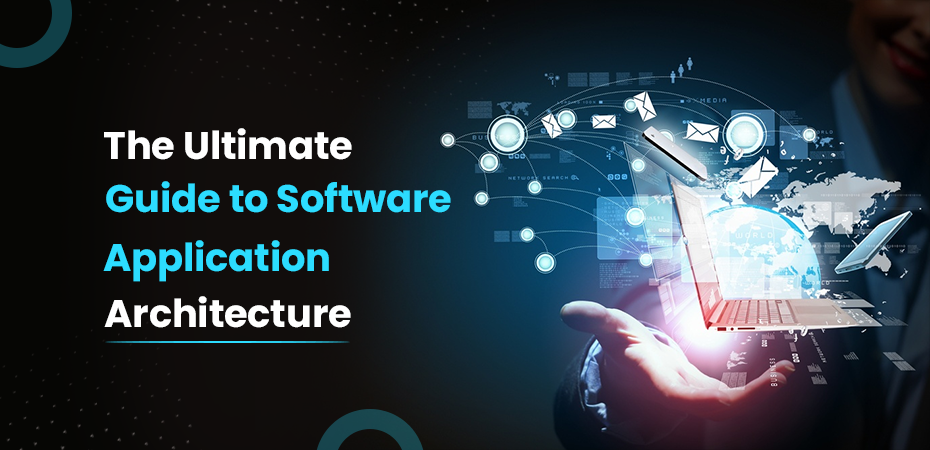
Understanding software application architecture is crucial for anyone involved in designing, developing, or managing software systems. But what exactly is software application architecture? It refers to the high-level structures of a software system and the discipline of creating such structures. This framework dictates how software components interact, how data flows, and how services are deployed.
Mastering application architecture or web app architecture offers many benefits. For one, it helps developers create scalable and maintainable applications. Additionally, it enables architects to make informed decisions that align with business goals and technical requirements.
In this comprehensive guide, we explore the various facets of application architecture:
- Understanding Architecture: Architecture meaning and concepts around monolithic, microservices, and serverless architectures.
- Design Patterns: Detailed look at MVC and other common patterns.
- Client-Server Model: Explanation of this fundamental model.
- Infrastructure Organization: Comparison between on-premises solutions and cloud application architecture platforms.
- Quality Attributes: Importance in architectural decisions.
- Best Practices: Principles for effective architecture design app.
- Architecture and Programming: How they influence each other.
This guide aims to equip you with the knowledge required to become an effective application architect.
Understanding Architecture: Definitions and Concepts
Monolithic Architecture
Monolithic architecture refers to a single, unified model for designing software applications. This mobile app architecture integrates all functionalities of an application into one cohesive unit.
Characteristics of Monolithic Applications
- Single Codebase: The entire architectural application is contained within a single codebase, making it easier to manage in terms of version control and collaboration.
- Unified Deployment: All components are deployed together; if one component needs an update, the whole application must be redeployed.
- Tight Coupling: Components are tightly interwoven, which can simplify interactions but makes it challenging to isolate and fix issues.
Advantages
- Simpler Development and Testing: Developers work within a single codebase, simplifying the development process. Testing is straightforward because all components are part of one deployable unit.
- Easier Deployment: Only one deployment pipeline is required, making the process less complex compared to other web app architecture diagrams.
- Performance: Inter-process communication is generally faster within a monolithic application since all components share the same memory space.
Disadvantages
- Scalability Issues: Scaling a monolithic application often means duplicating the entire application across multiple servers, leading to inefficiencies.
- Limited Flexibility: Updating or modifying a small part of the system requires redeploying the whole application, which can slow down release cycles.
- Maintenance Challenges: As the application grows, maintaining a large codebase can become cumbersome and error-prone.
Monolithic architecture offers simplicity and ease of management for smaller applications but presents challenges in scaling and flexibility as the application grows. Understanding its characteristics helps in making informed decisions tailored to project requirements.
Microservices Architecture
Microservices architecture is a significant change from the traditional monolithic approach. Instead of building an application as a single unit, it breaks it down into smaller, independent services that communicate with each other. Each service focuses on a specific function, allowing teams to work on different parts of the application simultaneously.
Benefits of Microservices
- Scalability: Services can be scaled horizontally, ensuring efficient resource utilization.
- Independent Deployment: Each service can be updated without affecting the entire system, reducing downtime and enabling continuous delivery.
- Flexibility in Technology Choices: Different services can use different tech stacks best suited for their tasks, optimizing performance and development speed.
By adopting the best architecture apps, organizations can achieve higher agility and resilience in their software systems.
Serverless Architecture
Serverless computing is a cloud computing model where the cloud provider dynamically manages the infrastructure needed to run your code. Unlike traditional hosting models, you don’t need to manage servers or worry about maintaining the hardware.
Advantages of Serverless Architecture
- Reduced Operational Complexity: By leveraging Backend as a Service (BaaS) and Function as a Service (FaaS), developers can focus on writing code without managing server infrastructure.
- Cost Efficiency: You only pay for the compute time your code consumes, which can result in significant savings.
- Scalability: Serverless platforms automatically scale applications in response to demand, making them ideal for unpredictable workloads.
- By understanding these concepts, one can appreciate the shift towards serverless models in modern application development.
Comparison of Architectural Types
Understanding the key differences between architecture app types is crucial. It is needed for selecting the right approach for your mobile application architecture. Let us discuss the advantages of architectural and design software.
1. Monolithic Architecture
- Single Unified Model: All components are interconnected and interdependent.
- Advantages: This architect app has simplified deployment, easier to develop for smaller teams.
- Disadvantages: Scalability issues, difficult to manage as the application grows.
2. Microservices Architecture
- Loosely Coupled Services: Each service can be developed, deployed, and scaled independently.
- Advantages: Improved scalability, flexibility in technology choices.
- Disadvantages: Increased complexity in management and communication between services.
3. Serverless Architecture
- Backend as a Service (BaaS) or Function as a Service (FaaS): Reduces operational complexity by offloading server management.
- Advantages: Cost efficiency, automatic scaling.
- Disadvantages: Limited control over the execution environment, potential vendor lock-in.
When to Choose One Over Another?
- Opt for monolithic architecture if you are building a small application with limited scope and scalability requirements.
- Choose microservices architecture when you need robust scalability and flexibility, especially useful for large-scale applications with diverse functionalities.
- Consider serverless architecture if you want to minimize operational overhead and focus on rapid deployment and development cycles.
Model-View-Controller (MVC) Pattern in Application Architecture Design Patterns
The Model-View-Controller (MVC) pattern is a foundational concept in software design. It divides an application into three interconnected components:
- Model: Represents the data and the business logic. It is responsible for managing the data of the application, responding to requests for information, and updating itself according to instructions.
- View: The user interface component that displays the data. It renders the model’s data and sends user input to the controller.
- Controller: Acts as an intermediary between Model and View. It handles user input, updates the model, and decides which view should be displayed.
Benefits of MVC
- Separation of Concerns: Each component has a distinct responsibility, making it easier to manage complexity.
- Improved Maintainability: Isolating different parts of the application simplifies debugging and updating code.
- Enhanced Testability: Components can be tested independently, ensuring more reliable applications.
This pattern is prevalent in web development frameworks such as Ruby on Rails, ASP.NET MVC, and Django.
Other Common Design Patterns in Application Architecture Design Patterns
Modularization and Maintainability
Modularization plays a crucial role in maintaining complex cloud services and software systems.
By breaking down an application into smaller, interchangeable modules, developers achieve:
- Enhanced Manageability: Smaller codebases are easier to manage and understand.
- Improved Reusability: Modules can be reused across different parts of the application or even in other projects.
- Simplified Testing: Isolated modules simplify unit testing, allowing for more robust and reliable software.
To illustrate, consider a web based application architecture. Instead of building a monolithic structure, developers can create distinct modules for user authentication, data processing, and UI rendering.
Additional Design Patterns
Beyond MVC, several other design patterns are influential in application development:
- Singleton Pattern: Ensures a class has only one instance and provides a global point of access to it. This is particularly useful for managing global application state.
- Factory Pattern: Defines an interface for creating objects but allows subclasses to alter the type of objects that will be created. This promotes flexibility and scalability.
- Observer Pattern: Establishes a subscription mechanism to notify multiple objects about any events that happen to the object they are observing. Useful in event-driven architectures.
These patterns help create website application architecture diagrams that clearly define interactions and dependencies within the system, aiding both development and maintenance processes. The choice of pattern often depends on specific requirements such as scalability, maintainability, or simplicity of an application architecture diagram for web applications.
Understanding the Client-Server Model
The client-server model is a fundamental concept in software application architecture. It describes a system where multiple clients request and receive services from a centralized server. This model enables efficient resource management and promotes scalability.
Key Components of the Client-Server Model
- Clients: These are the end-user devices or architecture design applications that request services or resources.
- Servers: Centralized systems that provide services or resources to multiple clients simultaneously.
- Requests and Responses: Clients send requests to servers, which process these requests and return appropriate responses.
The Importance of APIs in Client-Server Communication
APIs (Application Programming Interfaces) play a crucial role in enabling communication between clients and servers. They define how requests should be formatted, what data should be included, and how responses are structured.
Benefits of Using APIs
- Standardization: APIs create a standardized way for different systems to interact.
- Abstraction: They abstract the underlying complexity of server operations from the client.
- Security: APIs often include security measures such as authentication and encryption to protect data exchanges.
By leveraging APIs, developers can ensure seamless interaction between disparate systems, enhancing interoperability and flexibility within the client-server model.
Best Practices for Software Application Architecture Principles for Effective Design
Adhering to key principles is essential in ensuring robust software application designs. Here are some fundamental principles every architect should consider:
- Separation of Concerns: Divide the application into distinct sections, each addressing a specific concern. This promotes maintainability and simplifies the debugging process.
- Modularity: Structure the application into smaller, interchangeable modules. This aids in isolating changes and facilitates independent development and testing.
- Scalability: Design the architecture to handle growing amounts of work seamlessly. Implementing horizontal scaling techniques allows for easier scaling out by adding more instances rather than upgrading existing ones.
- Resilience: Ensure the system can recover from failures gracefully. Incorporate redundancy and failover mechanisms to maintain continuous operation.
- Performance Efficiency: Optimize resource usage to provide fast response times and efficient processing. Use caching strategies and optimize database queries to reduce latency.
- Security: Protect data and resources against unauthorized access and breaches. Implement authentication, authorization, and encryption protocols to safeguard sensitive information.
- Documentation: Maintain comprehensive documentation that details architectural decisions, design patterns, and system interactions. Good documentation supports ongoing maintenance and onboarding of new team members.
- Continuous Integration & Continuous Deployment (CI/CD): Automate testing and deployment processes to ensure rapid delivery of updates while maintaining high quality standards.
By integrating these principles into your design process, you can develop applications that are not only robust but also adaptable to evolving requirements.
Understanding the Connection Between Architecture and Programming
Software architecture is like a blueprint for building software. It defines how different parts of the software will work together. This blueprint influences how programmers write their code, organize it, and make changes to it.
How Software Architecture Shapes Programming?
- Code Structure: The way the software is designed (its architecture) determines how the code is organized. For example, if the software follows a monolithic architecture, all the code will be in one place. On the other hand, if it uses microservices architecture, the code will be split into smaller, independent parts.
- Team Collaboration: The architecture also affects how teams work together. With microservices, different teams can work on their parts of the software simultaneously. This is different from monolithic systems where one team has to finish their work before another team can start.
- Scaling: The design choices made in the architecture impact how easily the software can grow or handle more users. Microservices allow individual parts of the application to be scaled independently, while monolithic applications may require a complete redeployment for scaling.
- Code Maintenance: A well-thought-out architecture makes it easier to maintain and update the code. Design patterns like MVC (Model-View-Controller) help in organizing code logically, making it simpler to fix bugs and add new features.
How Programming Choices Influence Software Architecture?
- Language and Framework Selection: The programming languages and frameworks used can affect which architectural styles are practical. For instance, languages that support concurrent programming are ideal for implementing microservices.
- Coding Standards: Maintaining high-quality coding standards ensures that the architectural vision is implemented effectively. If developers follow poor coding practices, it can lead to technical debt and compromise the integrity of the architecture.
- Adapting to Change: As new programming techniques emerge; architects need to adjust their designs accordingly. For example, serverless architectures can take advantage of modern event-driven programming approaches.
Having a clear understanding of how software architecture and programming influence each other is crucial for creating flexible and resilient applications.
Conclusion
Becoming skilled in software application architecture involves both learning and hands-on experience. With a grasp of different architectural styles, design patterns, and infrastructure approaches, you’ll be equipped to create strong and adaptable systems. Apply these ideas in your work, always improving your abilities to become a proficient architect who can design top-notch software solutions.