Build Faster Frontends with Vite JS
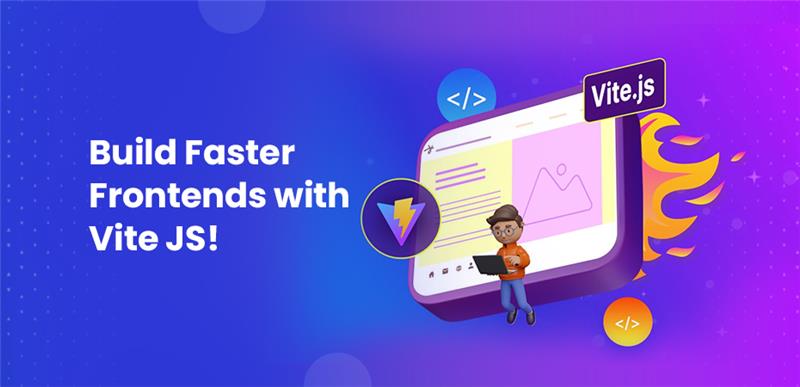
Have you ever felt frustrated waiting for your development server to start? Introducing Vite.js: A Powerful Tool for Frontend Development. This powerful build tool is changing the way we create modern web applications by making the development process incredibly fast.
Speed is no longer a luxury in today’s web development environment; it is a necessity. Users expect instant loading times, smooth interactions, and seamless experiences. Developers need tools that can keep up with these demands while maintaining productivity.
In this guide, you’ll learn:
- How Vite.js improves frontend development
- The secrets behind Vite’s fast server starts
- Setting up your first Vite project
- Advanced features for optimizing apps
- Comparisons with other popular build tools
Whether you’re building complex React apps, Vue frontend projects, or simple JavaScript applications, Vite (Javascript) JS offers a fast, efficient approach. Let’s explore how this tool can boost your development workflow and help you create faster frontends.
Understanding Vite.js
Vite.js, developed by Evan You, creator of Vue.js, addresses slow server start and build times in large applications. It stands out in the JavaScript ecosystem by:
- Dev Server Performance: Serves code over native ES modules instead of bundling everything first.
- Smart Dependency Handling: Pre-bundles dependencies with esbuild, which runs 10-100x faster than JavaScript-based bundlers.
- Production Optimization: Uses Rollup for Vite’s production builds, ensuring lean output and excellent browser compatibility.
Traditional bundlers like Webpack require complex configuration files and process entire application bundles on startup. Let’s compare:
Feature | Webpack | Vite Bundler |
---|---|---|
Bundling method | Bundles everything upfront | On-demand file serving |
Development server start time | Slower | Near-instant |
Configuration complexity | Complex configuration needed | Zero-config for common setups |
Hot Module Replacement (HMR) | JavaScript-based HMR | Native ESM-based HMR |
Vite uses Node JS for builds and dependencies but speeds up development with native ES modules and esbuild.
Key Features of Vite.js
Vite.js offers powerful features that make it a game-changer for frontend development.
1. Instant Server Start
Vite.js achieves lightning-fast server startup by leveraging native ES modules. It immediately starts the dev server without bundling your entire application first. This means:
- No Initial Bundle: Your app launches in milliseconds
- On-Demand Compilation: Files are compiled only when requested
- Smart Caching: Compiled results are cached for even faster subsequent starts
2. Hot Module Replacement (HMR)
The built-in HMR system in Vite.js transforms your development workflow with precise updates:
javascript // Changes in this component export default function Button() { return Click me } // Will update instantly without losing application state
HMR updates only the changed parts of the app, keeping the rest intact and avoiding full page reloads during development.
3. Optimized Builds with Rollup
Production builds in Vite uses Rollup’s power for optimal output:
- Tree Shaking: Eliminates unused code automatically
- Code Splitting: Generates smaller, more efficient chunks
- Asset Optimization: Compresses and optimizes static resources
- Dynamic Imports: Supports lazy loading for improved performance
4. Native ESM Serving
Vite.js serves code as native ES modules during development:
javascript import { useState } from ‘react’ import styles from ‘./styles.module.css’
This approach provides:
- Faster Browser Parsing: Native module resolution
- Improved Vite’s Debugging: Clear source mapping
- Better Code Organization: Direct module dependencies
5. Caching Pre-built Dependencies
The intelligent caching system maximizes development speed:
- Pre-bundled Dependencies: Common dependencies are pre-processed
- Browser-level Caching: Cached modules persist between sessions
- Smart Invalidation: Cache updates only when dependencies change
- bash
Example of dependency pre-bundling
vite optimize –force
Framework Support and Integration in Vite.js
Vite.js stands out with its wide range of framework support, providing ready-to-use templates for popular JavaScript frameworks. Let’s take a closer look at the main frameworks and how they integrate with Vite.js:
React Integration
- Zero-config TypeScript support
- Fast refresh implementation
- JSX and TSX support
- Automatic CSS modules
- Built-in PostCSS integration
Vue.js Support
If you’re a Vue.js developer, it’s incredibly straightforward to start Vue projects with Vite. Simply use the Vue plugin provided by the Vite team to enable Single File Components (SFC), template hot reloading, and scoped CSS.
- Vue 3 Single File Components (SFC)
- Template hot reloading
- Scoped CSS
- Custom blocks support
- TypeScript integration
Svelte Development
- Native component hot reloading
- Preprocessor support
- SCSS/Less integration
- TypeScript compatibility
- Optimized build output
Each framework integration offers specific performance benefits:
- Faster Development Cycles: Framework-specific hot module replacement reduces development time
- Optimized Production Builds: Framework-aware code splitting and tree-shaking
- Enhanced Developer Experience: Framework-specific plugins and tooling support
- Simplified Configuration: Pre-configured templates for quick project setup
- Improved Build Performance: Framework-specific optimizations for faster compilation
Vite framework integrations uphold the tool’s core principles of speed and efficiency while offering features that developers expect in their daily workflow. These integrations make Vite.js a flexible choice for teams using various frontend technologies.
Setting Up a Project with Vite.js
Let’s create your first Vite.js project with these simple steps:
1. Initialize Your Project
bash npm create vite@latest my-vite-app
2. Select Your Framework
- Choose from React, Vue, Svelte, or Vanilla JS
- Pick your preferred variant (JavaScript/TypeScript)
3. Navigate and Install Dependencies
bash cd my-vite-app npm install
4. Start Development Server
bash npm run dev
Project Structure
Your Vite’s new project includes these essential files:
my-vite-app/ ├── public/ # Static assets ├── src/ # Source code │ ├── assets/ # Project assets │ ├── components/ # UI components │ └── App.jsx # Root component ├── index.html # Entry point ├── package.json # Dependencies └── vite.config.js # Vite configuration
The vite.config.js file allows you to customize your build settings, plugins, and development server options. The public directory stores static assets that won’t require processing, while the src folder contains your application’s source code.
Exploring Plugins and Integrations for Enhanced Functionality
Vite’s plugin system provides extensive opportunities for customizing the development workflow. Let’s dive into some essential plugins that can supercharge your Vite projects.
Official Framework Plugins
1. Vue Plugin
To install the official Vue plugin, run the following command:
bash npm install @vitejs/plugin-vue
This plugin enables various features for Vue applications, including:
- Support for Single File Components (SFC)
- Handling of custom blocks in Vue files
- Optimization of template compilation process
- Hot Module Replacement (HMR) functionality for Vue components
2. React Plugin
To install the official React plugin, run the following command:
bash npm install @vitejs/plugin-react
This plugin provides several benefits for React projects, such as:
- Built-in support for Fast Refresh, allowing instant updates during development
- Transformation of JSX syntax into JavaScript
- Automatic configuration of React runtime based on the Vite version used
- Performance optimizations specifically targeted at development mode
Streamlining Your Workflow
Plugins in Vite projects can automate common tasks:
1. Style Processing
- Use vite-plugin-windicss to integrate utility-first CSS framework Windi CSS into your project.
- Employ @vitejs/plugin-legacy to ensure CSS compatibility with older browsers by generating vendor-prefixed styles.
2. Asset Handling
- Optimize images in your application using vite-plugin-imagemin to reduce file size without compromising quality.
- Load SVG files as React components or Vue components by utilizing vite-svg-loader.
3. Development Tools
- Inspect and debug build processes with vite-plugin-inspect to gain insights into how Vite bundles your application.
- Add Progressive Web App (PWA) capabilities to your project by leveraging vite-plugin-pwa.
Adding Plugins to Your Vite Config
Integrating plugins into your Vite configuration is a simple process. Here’s an example of how you can add both Vue and React plugins:
- javascript // vite.config.js import vue from ‘@vitejs/plugin-vue’ import react from ‘@vitejs/plugin-react’
- export default { plugins: [ vue(), react() ] }
Optimizing Front-end Development Using Advanced Features
Vite.js takes frontend development speed to the next level with its advanced features, particularly in TypeScript support and code-splitting capabilities.
Lightning-Fast TypeScript Integration
Vite’s TypeScript support through esbuild delivers remarkable compilation speeds:
- 20-30x faster compilation compared to traditional TypeScript compilers
- Zero configuration required – TypeScript works out of the box
- Built-in type checking during development
- Automatic .ts and .tsx file handling
typescript // Example: TypeScript in Vite interface User { name: string; age: number; }
const user: User = { name: “John”, age: 30 }
Smart Code-Splitting
Vite’s code-splitting strategy optimizes application performance through intelligent chunking:
- Dynamic Imports: Automatically splits code based on import statements
- Route-Based Splitting: Creates separate chunks for different routes
- On-Demand Loading: Loads modules only when needed
javascript // Example: Dynamic imports in Vite const AdminPanel = () => import(‘./components/AdminPanel.vue’)
Performance Benefits
The combination of esbuild and code-splitting delivers tangible improvements:
- Reduced initial bundle size
- Faster page loads
- Improved caching efficiency
- Better resource utilization
With its instant server start and efficient production builds, the Vite bundler outperforms traditional bundlers like Webpack in terms of speed and simplicity. Its ability to handle complex TypeScript projects while maintaining fast build times sets it apart from traditional build tools.
Comparative Study Between Popular Build Tools And Their Prospective Use Cases
Let’s dive into a detailed comparison of today’s most popular build tools to help you make informed decisions for your projects.
Vite vs. Webpack: Configuration and Performance
Configuration Complexity
- Webpack requires extensive configuration files with multiple loaders and plugins
- Vite offers zero-config setups with sensible defaults
- Webpack has a steeper learning curve due to its complex architecture
- Vite’s configuration is minimal and intuitive
Build Performance
- Webpack bundles all modules during development
- Vite leverages native ES modules for faster development builds
- Cold start times: Webpack (~20s) vs. Vite (~300ms)
- HMR speed: Webpack (~200ms) vs. Vite (~50ms)
When comparing tools, some developers suggest that Webpack is next better than Vite for projects requiring intricate customizations, given its extensive plugin ecosystem and well-established community.
Create React App vs. Vite: Developer Experience
Setup and Installation
Create React Applications:
- Installation time: 1-2 minutes
- Project size: ~200MB node_modules
- Predefined project structure
Vite:
- Installation time: 15-30 seconds
- Project size: ~50MB node_modules
- Flexible project structure
Development Experience
Create React App:
- Slower development server startup
- Limited customization without ejecting
- Built-in testing setup
Vite:
- Lightning-fast server startup
- Easy customization through plugins
- Framework-agnostic approach
Use Case Recommendations
Choose Webpack when:
- Working with legacy applications
- Need specific custom configurations
- Require extensive code transformations
Choose Vite when:
- Building modern applications
- Speed is a priority
- Need quick project iterations
Choose Create React App when:
- Starting a React-only project
- Prefer an opinionated setup
- Need built-in testing infrastructure
Conclusion
Vite.js is a game-changing tool in frontend development, offering fast server starts, efficient Hot Module Replacement, and optimized builds. With native ESM serving, smart dependency caching, and strong framework support, Vite.js is perfect for rapid development and large-scale builds.
It’s an ideal choice for teams focused on improving workflow efficiency. Ready to transform your frontend development? Build Offshore Team specializes in using Vite.js and other cutting-edge tools to create high-performance web applications for your next project.
Frequently Asked Questions (FAQs)
Yes, you can migrate existing projects to Vite.js, though it might require some configuration adjustments based on your project structure.
Vite produces builds compatible with browsers supporting ES modules. For older browsers, you can configure polyfills through plugins.
Yes, Vite.js handles large applications efficiently through its optimized build process and code-splitting features.
Developers familiar with modern JavaScript tools will find Vite.js easy to learn. Its straightforward configuration and extensive documentation make it accessible for beginners.
Yes, Vite.js supports commonly used CSS preprocessors such as Sass, Less, and Stylus through its built-in features or plugins.