C# vs Java: Which Language Reigns Supreme?
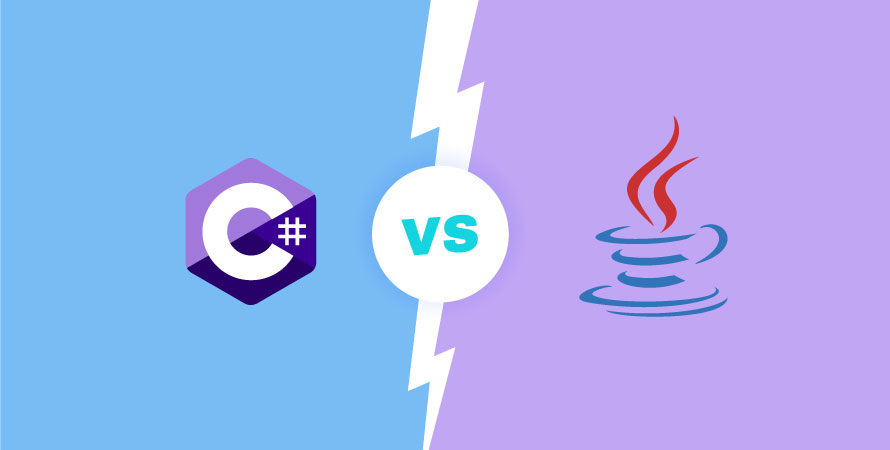
In the world of software development, it’s crucial to choose the right programming language. Two of the most popular programming languages are C# and Java.
The language C#, created by Microsoft in 1999, is a high-level, type-safe, object-oriented programming language that runs on the .NET Framework. It offers many features like versioning, virtual/override modifiers, and overload resolution rules.
Java programming language, developed by James Gosling for Sun Microsystems in 1995, is famous for its ability to “write once, run anywhere.” It can be used for a wide range of applications such as mobile apps, cloud computing, big data, and IoT development.
Comparing C# vs Java is important because they are widely used in various industries. Both languages have had a significant impact on modern software development practices, influencing everything from large-scale solutions to mobile apps. This comparison will help developers make better choices based on their project requirements and skills.
Programming languages like C# and Java are essential tools in software development. They not only determine how developers write code but also affect the performance, scalability, and maintainability of applications. Analysing Java vs C# can have a big impact on project success, so it’s crucial to understand their differences thoroughly.
Here, in this blog, you will learn about what is the C# language.
Background Information
History, Features, and Usage of C#
C# is a high-level, type-safe, object-oriented programming and compiled language developed by Microsoft in 1999. Initially conceptualized as part of the .NET initiative, C# was designed to be a simple, modern, and versatile language that could cater to a wide range of development needs.
Key Features of C#
- Object-oriented programming (OOP): Emphasizes encapsulation, inheritance, and polymorphism.
- Component-oriented programming: Encourages the creation of reusable software components.
- Automatic garbage collection: Manages memory efficiently by automatically reclaiming unused memory.
- Rich library support: Extensive .NET framework libraries enhance functionality.
- Language interoperability: Allows seamless integration with other .NET languages.
Usage of C#
C# finds extensive usage across various domains:
- Enterprise applications: Widely used for developing business applications due to its robustness and integration capabilities.
- Web development: ASP.NET allows developers to build dynamic websites and web applications.
- Game development: Unity, a popular game engine, leverages C# for scripting.
- Mobile applications: Xamarin enables cross-platform mobile app development using C#.
History, Features, and Usage of Java
Java development language, created in May 1995 by James Gosling at Sun Microsystems, emerged as a groundbreaking language emphasizing platform independence. Known for its “write once, run anywhere” philosophy, Java quickly gained traction in various fields.
Key Features of Java
- Platform independence: The JVM (Java Virtual Machine) allows software Java programs to run on any device with a compatible JVM.
- Robust memory management: Automatic garbage collection ensures efficient memory usage.
- Security features: Built-in security mechanisms protect against common vulnerabilities.
- Multithreading support: Facilitates concurrent execution of multiple threads.
- Extensive standard library: Provides ready-to-use classes for various functionalities.
Usage of Java
Java is a highly versatile language. This versatility makes it suitable for numerous applications:
- Mobile apps: Dominates Android app development through Android Studio.
- Enterprise solutions: Utilized in large-scale enterprise systems due to their scalability and security.
- Web applications: Frameworks like Spring and Hibernate streamline web development processes.
- Cloud computing and big data: Supports cloud-based applications and big data technologies like Hadoop.
Syntax and Language Features
Syntax Comparison Between C# and Java
C# and Java have a similar syntax, making it easy for developers to switch between them. These widely used programming languages use C-style syntax, including curly braces {} for code blocks and semicolons; to end statements. You might be thinking is C# similar to Java or not. However, there are some subtle differences in Java or C sharp language:
Example of a simple class in C#:
csharp using System;
public class HelloWorld { public static void Main(string[] args) { Console.WriteLine(“Hello, World!”); } }
Example of a simple class in Java:
java public class HelloWorld { public static void main(String[] args) { System.out.println(“Hello, World!”); } }
In C#, the Main method is defined with an uppercase ‘M’, while in Java, it is lowercase ‘m’. Additionally, the using keyword in C# corresponds to the import statement in Java for including libraries.
Differences in Language Features Between C# and Java
While their syntax might be similar, Java versus C sharp have differences in their language features that affect how developers write and manage code.
Properties vs Getters/Setters
C# uses properties that simplify the syntax for defining getters and setters.
C# Example:
csharp public class Person { public string Name { get; set; } }
Java Example:
java public class Person { private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
LINQ (Language Integrated Query)
C# offers LINQ for querying collections in a readable manner. This feature allows developers to write queries directly within the language using syntactic sugar.
C# LINQ Example:
csharp int[] numbers = { 1, 2, 3, 4, 5 }; var evenNumbers = numbers.Where(n => n % 2 == 0).ToList();
Java does not have an equivalent feature built into the language itself but can achieve similar functionality using streams introduced in Java 8.
Java Streams Example:
java int[] numbers = { 1, 2, 3, 4, 5 }; List evenNumbers = Arrays.stream(numbers) .filter(n -> n % 2 == 0) .boxed() .collect(Collectors.toList());
Memory Management
Both languages provide automatic garbage collection but handle memory management differently. C# includes features like the ref and out keywords, which allow passing arguments by reference.
C# Example:
csharp public void Increment(ref int number) { number++; }
Java does not support passing by reference explicitly but allows modifying objects passed as parameters since object references are passed by value.
Pointers and Unsafe Code
C# allows the use of pointers within an unsafe context for scenarios demanding high-performance operations that require direct memory access.
C# Unsafe Example:
csharp unsafe { int x = 10; int* p = &x; Console.WriteLine((int)p); }
Java does not support pointers directly due to its design principle emphasizing security and robustness.
Exception Handling
Both languages support exception handling with try-catch blocks but differ slightly in their approach to checked exceptions. Java enforces checked exceptions at compile-time requiring explicit handling or declaration using the throws keyword. In contrast, C# only has unchecked exceptions (runtime exceptions).
Understanding these syntactic similarities and differences is crucial for developers working across both languages or deciding which suits their project requirements better. Analyzing Java programming vs C++ makes the development work easier for the software developers.
Development Tools and Frameworks
Overview of Microsoft Visual Studio for C# Development
Microsoft Visual Studio is one of the most comprehensive integrated development environments (IDEs) for C#. It offers:
- .NET Framework: Smooth integration with .NET Framework, making it easier to develop web, desktop, and mobile applications.
- IntelliSense: Advanced code completion features that help in writing code more efficiently.
- Debugging Tools: Strong debugging capabilities, including breakpoints, watch windows, and immediate windows for real-time code execution analysis.
- Version Control: Built-in support for Git and other version control systems.
- Extensions: A wide range of extensions to enhance functionality.
Overview of Android Studio for Java Development
Android Studio, the official IDE for Android app development, provides a rich set of tools designed specifically for Java developers:
- Gradle-Based Build System: Efficient project management and build automation.
- Layout Editor: Drag-and-drop interface design with real-time preview on multiple screen configurations.
- APK Analyzer: Tool to analyze the size and composition of APK files, optimizing application performance.
- Advanced Code Editor: Code completion, refactoring, and linting to ensure best practices.
- Emulator: Built-in Android emulator providing a complete environment to test applications across various device configurations.
Comparison of Development Tools Available for C# and Java
Both C# and Java have strong ecosystems supported by powerful development tools. Here’s a comparison:
| Feature/Tool | C# (Microsoft Visual Studio) | Java (Android Studio) | |————————|——————————|——————————-| | IDE | Microsoft Visual Studio | Android Studio | | Build System | MSBuild | Gradle | | Debugging | Advanced | Advanced | | Code Completion | IntelliSense | Smart Code Completion | | Platform Integration | .NET Framework | Android SDK | | Device Emulation | Limited | Extensive |
Visual Studio’s deep integration with the .NET ecosystem is ideal for enterprise-level application development. On the other hand, Android Studio is unmatched in its specialized tools for mobile app development on the Android platform.
Exploring these tools reveals how both languages are supported by mature and feature-rich environments tailored to their respective strengths.
Application Areas
Application Areas Where C# is Commonly Used
C# has carved out a significant niche in various domains, driven by its integration with Microsoft’s .NET Framework:
Enterprise Software
Many large-scale enterprise applications leverage C# due to its robust framework and comprehensive toolset. Companies such as Stack Overflow and Bank of America use C# for their backend systems.
Web Development
ASP.NET, a framework built on C#, allows developers to create dynamic web applications and services. It provides high performance and scalability, making it suitable for large web projects.
Game Development
Unity, one of the most popular game engines, uses C# as its primary scripting language. This has made C# a go-to language for game developers.
Windows Applications
Given its roots in Microsoft, C# is extensively used for developing Windows desktop applications.
Application Areas Where Java is Commonly Used
There are plenty of application areas where is Java still used. Java’s platform independence and extensive libraries make it a versatile language:
Android Development
Java has been the cornerstone of Android app development for years, providing a robust environment for mobile applications.
Enterprise Software
Java’s scalability and reliability make it ideal for enterprise-level applications. Companies like Google and Amazon rely heavily on Java-based systems.
Web Development
With frameworks like Spring and Hibernate, Java offers powerful solutions for building complex web applications.
Big Data Technologies
Java is often used in big data platforms such as Apache Hadoop due to its performance and reliability.
Advantages and Disadvantages
Advantages of Using C# for Software Development
- Integration with Microsoft Ecosystem: C# seamlessly integrates with other Microsoft products like Azure, SQL Server, and Windows, making it an excellent choice for enterprise solutions.
- Rich Library Support: The .NET framework provides a vast library that simplifies complex tasks such as file manipulation, database interaction, and web services.
- Memory Management: Automatic garbage collection helps in efficient memory management, reducing the chances of memory leaks.
- Language Features: Supports modern programming paradigms including generics, lambda expressions, and asynchronous programming with async/await.
Advantages of Using Java for Software Development
- Platform Independence: Java’s “write once, run anywhere” capability ensures that applications can run on any device with a JVM installed.
- Robust Security Features: Built-in security features make Java suitable for networked applications where security is a concern.
- Large Community and Ecosystem: Extensive support from a large developer community and a rich ecosystem of libraries and frameworks like Spring, Hibernate, and Apache Struts.
- Scalability: Well-suited for building scalable applications ranging from mobile apps to large-scale enterprise systems.
Disadvantages or Limitations of C#
- Platform Dependency: Primarily designed to run on the Windows platform; while .NET Core allows cross-platform development, it’s not as mature as JVM.
- Learning Curve: New developers may find the learning curve steep due to its extensive features and integrations.
- Resource Intensive: Applications built with C# can be resource-intensive, requiring substantial system resources to run efficiently.
Disadvantages or Limitations of Java
- Performance Overhead: Java applications often have higher memory consumption and slower startup times compared to native applications.
- Verbosity: Writing code in Java can be more verbose compared to other languages which can lead to longer development times.
- GUI Development: Developing graphical user interfaces in Java can be cumbersome compared to other languages like Python or C#.
Both languages offer unique strengths and face individual challenges. The choice between C# and Java often depends on specific project requirements, existing infrastructure, and developer expertise.
Conclusion
When deciding between C# vs Java, the choice largely depends on specific project requirements and personal or organizational preferences. Each general-purpose programming language has its strengths. C# offers robust memory management and seamless integration with Windows environments. Java stands out with its platform independence and extensive use in mobile and web applications.
The decision between C# and Java should be guided by the specific needs of your project, existing infrastructure, and long-term goals. Both languages promise a bright future, backed by strong community support and continuous updates.