Common Problems JavaScript Developers Face
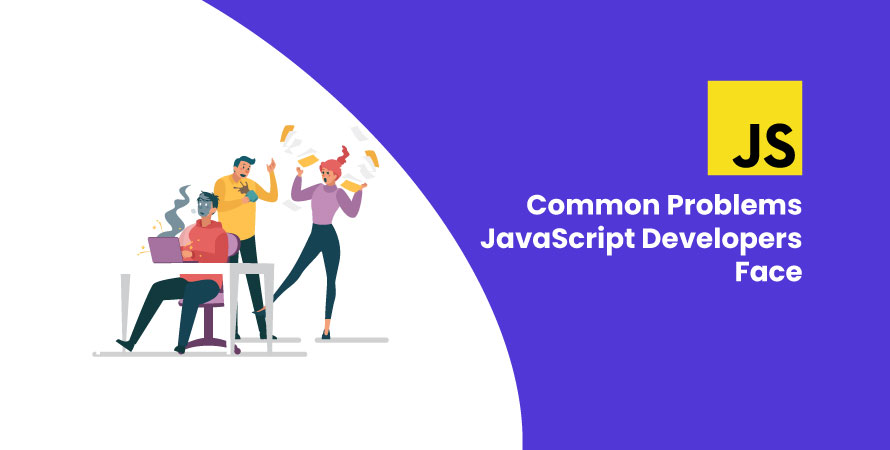
JavaScript developers often face various common problems that can affect their coding experience and the functionality of their applications. Understanding these common JavaScript errors is crucial for improving coding practices and creating more efficient, maintainable code.
Dealing with these issues directly helps developers avoid mistakes that can cause bugs, slow performance, and complicated debugging situations. By spotting and fixing common Java Script errors early in the development process, programmers can greatly boost both their productivity and the quality of their software.
This article explores several key challenges faced by JavaScript developers:
- Syntax Errors: Recognizing and fixing fundamental coding mistakes.
- Performance Issues: Understanding how inefficient code can slow down applications.
- Confusion between Assignment and Comparison Operators: Avoiding logical errors in javascript with proper operator usage.
- Understanding Scope in JavaScript: Managing variable accessibility effectively.
- This Keyword Misunderstandings: Handling context correctly to prevent unexpected behavior.
- Asynchronous Programming Challenges: Efficiently managing asynchronous operations.
- Strict Mode Confusion and Memory Management Issues: Utilizing strict mode properly and avoiding memory leaks.
- Type Coercion Issues, Inefficient DOM Manipulation, Error Handling Practices: Handling data types, DOM operations, and errors efficiently.
By discussing these topics, this article aims to provide JavaScript developers with practical strategies to overcome common challenges, leading to better coding practices and improved application performance.
Syntax Errors
Syntax errors are common issues that JavaScript developers encounter. They occur when the code violates the rules of the JavaScript language, preventing scripts from executing correctly.
Java Scripting Examples
Syntax js errors can take different forms:
- Missing or misplaced characters: Forgetting to close a parenthesis or misplacing a semicolon.
javascript function greet(name { console.log(“Hello, ” + name); }
- Incorrect keywords: Using a reserved keyword improperly.
javascript var for = “loop”;
- Typographical mistakes: Misspelling a variable name or function.
javascript consoel.log(“Hello, World!”);
Common Causes
Several factors contribute to syntax errors:
- Typos and carelessness: Simple mistakes in typing can lead to syntax issues.
- Lack of understanding: Not fully grasping JavaScript’s syntax rules and conventions.
- Complex codebases: Working with large amounts of sample js code increases the likelihood of mistakes.
Strategies to Identify and Fix Syntax Errors Quickly
Identifying and fixing syntax errors efficiently is crucial for maintaining smooth development workflows:
- Use linters: Tools like ESLint automatically detect syntax js check undefined errors and suggest corrections. bash npm install eslint –save-dev
- Code editors with built-in error detection: Modern IDEs such as VSCode highlight syntax errors as you type.
- Break down complex code: Simplify large functions into smaller, manageable pieces to minimize errors.
- Regular testing: Running frequent tests helps catch syntax mistakes early.
By recognizing common js causes and employing effective strategies, developers can reduce the frequency and impact of syntax errors, ensuring smoother coding experiences.
Performance Issues
Performance issues inside JavaScript often stem from poor coding practices, leading to slow application performance. Inefficient code can cause a range of problems, particularly when handling large datasets or complex operations.
Impact of Inefficient DOM Manipulation
Inefficient manipulation of the Document Object Model (DOM) is a common culprit behind sluggish applications. Directly manipulating the DOM frequently can result in:
- Reflows and Repaints: Each time the DOM is updated, the browser must recalculate the layout and redraw the page. This process, known as reflow and repaint, can be costly in terms of performance.
- Long Task Execution: Extensive DOM operations can block the main thread, causing long tasks that lead to unresponsive user interfaces.
Techniques for Profiling and Optimizing JavaScript Code
To address these issues, developers need robust techniques for profiling and optimizing their JavaScript code:
Profiling Tools:
- Chrome DevTools: Utilize Chrome’s built-in tools to analyze performance bottlenecks and understand where time is being spent during execution.
- Lighthouse: An open-source tool integrated into Chrome DevTools that audits performance among other metrics.
Optimizing Tips:
- Debounce and Throttle Functions: Limit the rate at which functions are executed, particularly useful for event listeners.
- Batch DOM Updates: Use document.createDocumentFragment() to batch multiple updates into a single operation.
- Use Efficient Data Structures: Choose appropriate data structures that minimize overhead and improve access times.
Profiling helps identify areas needing optimization, while deliberate coding practices ensure sustained performance improvements. Addressing these common pitfalls not only enhances application efficiency but also improves user experience significantly.
Confusion between Assignment and Comparison Operators
Understanding the distinction between the assignment operator (=) and comparison operators (==, ===) is crucial for JavaScript developers to avoid logical errors.
Differences Between =, ==, and ===
- Assignment Operator (=): Used to assign a value to a variable. Example: let x = 5; assigns the value 5 to the variable x.
- Equality Operator (==): Compares two values for equality after performing type coercion if necessary. Example: 1 == ‘1’ evaluates to true because the string ‘1’ is coerced to a number before comparison.
- Strict Equality Operator (===): Compares both value and type without performing type coercion. Example: 1 === ‘1’ evaluates to false because a number is not strictly javascript string equals.
Common Pitfalls
Misusing these operators can lead to subtle bugs:
- Accidental Assignment: Mistaking an assignment for a comparison can inadvertently change variable values. Example: if (x = y) {} assigns the value of y to x, which always evaluates as true if y is truthy.
- Type Coercion Issues: Relying on the equality operator can cause unexpected results due to implicit type conversion. Example: ‘0’ == false evaluates to true, which may not be the intended logic.
Best Practices
To prevent such logical errors, consider these best practices:
- Use Strict Equality (===): Prefer using strict equality checks (===) over loose equality checks (==) to avoid unintended type coercion.
- Linting Tools: Utilize tools like ESLint to catch potential misuse of assignment and comparison operators in your codebase.
- Code Reviews: Regular code reviews can help spot these common mistakes early in the development process.
By paying attention to these differences and adopting best practices, developers can write more reliable and bug-free JavaScript code.
Understanding Scope in JavaScript
Scope in JavaScript defines the accessibility of variables and functions in different parts of the code. There are several types of scope:
Global Scope
Variables declared outside any function are in the global scope. They can be accessed from anywhere in the code.
javascript var globalVar = “I’m a global variable”;
function checkGlobal() { console.log(globalVar); // Accessible here }
checkGlobal(); console.log(globalVar); // Accessible here too
Local Scope
Variables declared within a function are in local scope. They are only accessible within javascript check for undefined function.
javascript function localScopeFunction() { var localVar = “I’m a local variable”; console.log(localVar); // Accessible here }
localScopeFunction(); console.log(localVar); // Error: localVar is not defined
Block-Level Scope
Introduced with ES6, let and const allow variables to have block-level scope. These variables exist only within the block they are defined.
javascript if (true) { let blockVar = “I’m a block-level variable”; console.log(blockVar); // Accessible here }
console.log(blockVar); // Error: blockVar is not defined
Incorrect references often lead to bugs, especially when variables with similar names exist in different scopes. For instance, using a global variable unintentionally inside a function can cause unexpected behavior.
Tips for Managing Scopes Effectively
- Use let and const: Prefer these over var to avoid accidental global declarations and ensure proper block-level scoping.
- Minimize Global Variables: Keep the global namespace clean by limiting the number of global variables.
- Function Encapsulation: Encapsulate related functionality within js undefined functions to maintain clear and manageable scopes.
- Consistent Naming Conventions: Use consistent naming conventions to differentiate between variables in different scopes.
Understanding and managing scope effectively helps prevent bugs and enhances code readability.
Misunderstandings about this Keyword
This keyword in JavaScript often confuses developers due to its dynamic nature. It behaves differently depending on where it is used, which can lead to unexpected results.
Understanding the Context of this
1. Global Context
When used in the global scope, this refers to the global object (window in browsers).
javascript console.log(this === window); // true
2. Function Context
Inside a function, this refers to the global object (window) if the function is called without an object context.
javascript function showThis() { console.log(this); } showThis(); // window (in browsers)
3. Method Context
In methods, this refers to the object that owns the method.
javascript const obj = { name: “JavaScript”, showName: function() { console.log(this.name); } }; obj.showName(); // JavaScript
Asynchronous Programming Challenges
Asynchronous programming is essential in modern web applications, enabling tasks to run concurrently without blocking the execution of other operations. This approach is crucial for enhancing performance, especially in I/O-bound tasks like network requests and file handling. JavaScript developers often use callbacks, promises, and async/await to manage asynchronous operations.
Common challenges:
- Callback Hell: Nesting multiple callbacks can create complex and hard-to-maintain code structures, often referred to as “callback hell.”
javascript fetchData(url, function(response) { processResponse(response, function(result) { saveResult(result, function(saved) { console.log(‘Data saved successfully’); }); }); });
- Unhandled Promise Rejections: Failing to handle promise rejections can lead to silent errors that disrupt application flow.
javascript fetchData(url) .then(processResponse) .then(saveResult) .catch(error => console.error(‘Error:’, error));
Best practices:
- Using Promises: Convert callback-based functions into promises to simplify chaining asynchronous operations.
javascript fetchData(url) .then(response => processResponse(response)) .then(result => saveResult(result)) .then(() => console.log(‘Data saved successfully’)) .catch(error => console.error(‘Error:’, error));
- Async/Await Syntax: Leverage async/await for more readable and maintainable asynchronous code.
javascript async function handleData(url) { try { const response = await fetchData(url); const result = await processResponse(response); await saveResult(result); console.log(‘Data saved successfully’); } catch (error) { console.error(‘Error:’, error); } }
- Error Handling: Ensure robust error handling in all asynchronous operations to prevent silent failures.
Managing asynchronous programming effectively enhances both the performance and reliability of applications, making it a vital skill for JavaScript developers.
Strict Mode Confusion and Memory Management Issues
Understanding Strict Mode in JavaScript
Strict mode in JavaScript is a way to enforce stricter parsing and error handling in your code. It aims to catch common.js coding errors and provide a safer, more optimized execution environment.
Purpose of Strict Mode:
- Catching Common Mistakes: By enabling strict mode, developers can identify silent errors that could lead to bugs.
- Preventing Unsafe Actions: It disallows certain actions that are considered unsafe or error-prone.
To enable strict mode, you simply add “use strict”; at the beginning of your JavaScript file or function:
javascript “use strict”; function myFunction() { // Function code here }
New Restrictions Introduced by Strict Mode
Strict mode introduces several restrictions that may confuse developers:
- Eliminates this Binding to Global Object: javascript function foo() { console log object object (this); // undefined in strict mode } foo();
- Prevents Accidental Globals: javascript “use strict”; var x = y = 10; // Throws an error because y is not declared
- Disallows Duplicate Parameter Names: javascript function sum(a, a) { // Syntax error in strict mode return a + a; }
Recommendations for Using Strict Mode Effectively
- Use it Consistently: Apply strict mode across all your files or functions.
- Understand its Rules: Familiarize yourself with the new restrictions to avoid confusion.
- Leverage IDEs and Linters: Tools like ESLint can help identify issues related to strict mode.
Tips for Avoiding Memory Management Pitfalls
- Remove Event Listeners When No Longer Needed: javascript element.removeEventListener(‘click’, handleClick);
- Avoid Unnecessary Global Variables: Keep the global namespace clean and use local variables whenever possible.
- Use WeakMap for Managing Object References: javascript const wm = new WeakMap(); let obj = {}; wm.set(obj, ‘someValue’);
obj = null; // Now ‘obj’ can be garbage collected.
Effective management of memory and understanding the implications of strict mode are crucial for writing efficient, bug-free JavaScript code. Addressing these issues helps enhance both performance and maintainability of applications.
Type Coercion Issues, Inefficient DOM Manipulation, Error Handling Practices
Understanding Type Coercion in JavaScript
Type coercion in JavaScript refers to the implicit conversion of values from one data type to another. This process can lead to unexpected results and bugs if not properly understood.
Potential Pitfalls:
- String and Number Conversion: When a string is used in a context expecting a number, JavaScript attempts to convert it, sometimes leading to surprising outcomes. javascript console.log(“5” – 1); // Outputs: 4 console.log(“5” + 1); // Outputs: “51”
- Boolean Coercion: Non-boolean values are often coerced into boolean values in conditional statements. javascript if (“”) { console.log(“This will not be printed”); }
Best Practices:
- Use Explicit Conversion: Convert types explicitly using functions like Number(), String(), and Boolean(). javascript let num = Number(“5”); // Converts string “5” to number 5
- Strict Equality (===): Always use strict equality checks to avoid unintended type coercion. javascript if (num === “5”) { console.log(“This will not be printed”); }
Efficient DOM Manipulation Techniques
DOM manipulation in JavaScript is essential for dynamic web applications. However, improper handling can lead to performance bottlenecks.
Performance Bottlenecks:
- Frequent DOM Access: Accessing and modifying the DOM repeatedly can slow down your application due to reflows and repaints.
- Direct Manipulation: Making multiple changes directly on the DOM increases rendering time.
Techniques for Efficient DOM Updates:
- Batch Updates Using Document Fragments: Group multiple changes together before updating the DOM. javascript let fragment = document.createDocumentFragment();
for (let i = 0; i < 1000; i++) { let div = document.createElement(‘div’); fragment.appendChild(div); }
document.body.appendChild(fragment);
- Minimize Reflows and Repaints: Use CSS classes or innerHTML to set multiple styles or content at once.
Robust Error Handling Practices
Robust error handling is crucial for maintaining stable applications and preventing silent failures during runtime or production environments.
Common Strategies:
- Try/Catch Blocks: Wrap code that might throw an error in try/catch blocks. javascript try { let result = riskyOperation(); console.log(result); } catch (error) { console.error(“An error occurred:”, error); }
- Custom Error Handling Functions: Centralize error handling logic.
Tools for Debugging and Monitoring Errors:
- Console Logging: Use console.error() and console.warn() for logging errors and warnings.
- Error Monitoring Services: Integrate tools like Sentry or New Relic to track errors in production environments.
With these practices, developers can handle common challenges more effectively, ensuring smoother development processes and better-performing applications.
Conclusion
Improving your JavaScript skills is essential for developing efficient, maintainable, and high-performance applications. By adopting best practices like robust error handling, efficient memory management, and understanding JavaScript’s quirks (such as scope and type coercion), you can prevent common issues. Staying updated with the latest trends, tools, and frameworks is equally important to ensure your code remains modern and optimized. Continuous learning through blogs, forums, and conferences helps you stay ahead in the rapidly evolving JavaScript ecosystem.
Looking for expert support? Partner with Build Offshore Team to elevate your JavaScript projects with top-tier development talent.