Creating Custom Widgets in Flutter: Extending Functionality
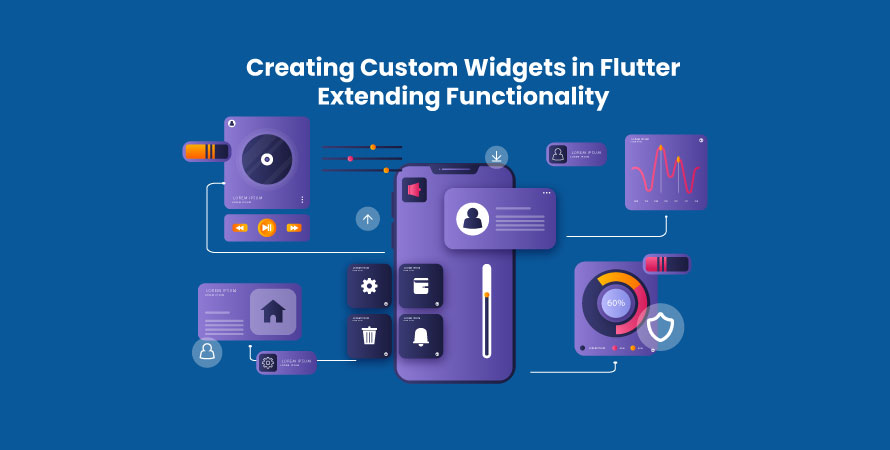
Creating custom widgets in Flutter is an essential skill for any app developer. It is basically for developers who are looking to build a unique and dynamic user interface. Custom widgets are user-defined UI components that allow developers to encapsulate specific functionalities, interactions, and designs. This capability not only enhances the visual appeal of the application but also optimizes performance and usability.
Custom Flutter widgets offer several advantages:
- Tailored Functionality: Developers can create widgets that meet the specific needs of their application, enabling more complex UI elements and interactions.
- Reusability: By defining custom widgets, code duplication is minimized, streamlining development workflows and making it easier to maintain the codebase.
- Consistent UI/UX Design: Custom widgets help achieve a consistent look and feel across the app, contributing to a better overall user experience.
These benefits highlight why creating custom widgets is crucial for extending the functionality of your Flutter applications. As you delve deeper into this topic, you’ll discover how to add custom widgets on Android, integrate them effectively into your projects, and optimize them for performance.
The journey of mastering custom widgets will unlock new possibilities in your app development endeavors. This allows you to craft truly unique and compelling mobile experiences. Here, in this blog, you will learn how to make custom widgets.
Understanding the Role of Custom Widgets in Flutter
In the world of Flutter components, custom widgets in Android are UI components created by developers. These are developed to meet specific requirements that pre-built widgets may not fulfill. These custom widgets represent unique aspects of an app’s functionality and appearance, offering tailored solutions for specific design needs.
Purpose and Benefits of Custom Widgets
Purpose:
- Customization: Custom widgets allow developers to create unique UI elements that match the app’s branding and user experience objectives.
- Reusability: By building reusable components, you can maintain consistency throughout different parts of your application.
- Encapsulation: They help encapsulate complex functionalities, making the codebase more modular and easier to manage.
Benefits:
- Unique UI/UX Design: Custom widgets play a significant role in creating a distinctive and cohesive UI/UX design, setting your app apart from others.
- Streamlined Workflow: They reduce code duplication by enabling you to reuse components across multiple screens or projects.
- Improved Performance: Well-designed custom widgets can optimize specific interactions and rendering processes, resulting in better performance.
Creating custom widgets in Flutter allows developers to expand the framework’s capabilities, leading to more dynamic and responsive applications. This flexibility is one of the main reasons why Flutter is a popular choice for modern mobile app development.
Building Your Custom Widgets in Flutter
Understanding Stateful and Stateless Widgets
Flutter sizedbox offers two main types of widgets:
- Stateful Widgets: These are dynamic widgets that can change their appearance based on user interactions or other factors. Use stateful widget building blocks when you need to manage a widget’s state over time.
- Stateless Widgets: These are static widgets that do not change once built. They are ideal for displaying fixed information.
Step-by-Step Process for Building Custom Widgets
Defining the Widget’s Purpose and Desired Behavior
To start, clearly define what you want your custom widget to achieve. For example, creating a reusable button with specific animations or a custom layout component.
Constructing the Widget
Using either stateful or stateless classes depends on your widget’s requirements.
Example: Creating a Stateless Widget
dart class CustomStatelessWidget extends StatelessWidget { final String text;
CustomStatelessWidget({required this.text});
@override Widget build(BuildContext context) { return Text(text); } }
Example: Creating a Stateful Widget
dart class CustomStatefulWidget extends StatefulWidget { @override _CustomStatefulWidgetState createState() => _CustomStatefulWidgetState(); }
class _CustomStatefulWidgetState extends State { bool isPressed = false;
void togglePressed() { setState(() { isPressed = !isPressed; }); }
@override Widget build(BuildContext context) { return GestureDetector( onTap: togglePressed, child: Container( color: isPressed ? Colors.blue : Colors.red, child: Text(‘Press me’), ), ); } }
Implementing Interactivity Logic
Incorporate logic to handle user interactions within your widget. As, responding to taps or gestures using Flutter’s GestureDetector.
Testing Strategies
Ensure optimal performance and reliability by:
- Running unit tests to verify the functionality.
- Using integration tests to check how the widget interacts within the app.
- Profiling the widget for performance bottlenecks using Flutter DevTools.
Creating custom widgets enhances the flexibility and reusability of your app components, ensuring a unique and consistent user experience.
Strategies for Effective Integration of Custom Widgets into Flutter Apps
Code Reusability with Custom Widgets
Custom widgets are essential for reusing code effectively. The developers can easily use these components in different parts of the app by creating custom widgets that contain specific functionalities and designs. This not only makes the development process smoother but also ensures consistent behavior and appearance, saving time and effort.
Enhancing User Experience
You can add custom UI elements like animations and gesture support to build Android widgets to make the user experience better. Flutter keys provide a wide range of libraries that allow you to create complex animations that grab users’ attention. For example, using packages like flutter_animations, you can create smooth transitions and interactive elements that improve the overall look and feel of your app.
dart class AnimatedButton extends StatefulWidget { @override _AnimatedButtonState createState() => _AnimatedButtonState(); }
class _AnimatedButtonState extends State with SingleTickerProviderStateMixin { AnimationController _controller;
@override void initState() { super.initState(); _controller = AnimationController( duration: const Duration(seconds: 2), vsync: this, )..repeat(reverse: true); }
@override Widget build(BuildContext context) { return ScaleTransition( scale: _controller, child: ElevatedButton( onPressed: () {}, child: Text(“Click Me”), ), ); }
@override void dispose() { _controller.dispose(); super.dispose(); } }
Handling User Interaction
It’s important to handle user interaction effectively within custom widgets to ensure responsive design. You can use event handling mechanisms like GestureDetector or InkWell to detect user actions such as taps, swipes, and drags. These interactions make sure that the widget responds smoothly to user input, creating a seamless experience.
dart GestureDetector( onTap: () { print(“Widget tapped!”); }, child: Container( color: Colors.blue, height: 100, width: 100, ), )
Incorporating these strategies into your Flutter apps allows for more dynamic and engaging user interfaces while maintaining efficient and clean code practices.
Refactoring and Optimizing Tips for Well-Maintained Custom Widgets
Reducing Code Duplication
Identifying and reducing code duplication is essential for maintaining clean and efficient custom widgets. Abstraction and composition techniques are effective strategies to achieve this goal:
Abstraction
Extract common functionalities into separate methods or classes. This minimizes repetitive code, making the widget more modular and easier to manage.
Composition
Combine smaller, reusable widgets to build complex UI components. This approach promotes reusability and simplifies the overall widget structure.
Monitoring Performance Metrics
Performance optimization is crucial for creating smooth and responsive custom widgets. Flutter quantity widget provides tools to monitor performance metrics, ensuring optimal widget behavior:
- Use the shouldRebuild method, which serves a similar purpose as shouldComponentUpdate() in React. This method helps control when a widget should be rebuilt. It ultimately optimizes rendering performance.
- Leverage the built-in Flutter DevTools to analyze and track performance issues. Identifying bottlenecks allows developers to make informed decisions about necessary optimizations.
Importance of Thorough Documentation
Thorough documentation is vital for the usability and maintainability of Android custom widgets. Each custom widget should include:
- Usage Guide: Clear instructions on how to integrate the widget into different parts of the app.
- Potential Caveats: Highlight any known limitations or special considerations to avoid unexpected issues during implementation.
- Example Code: Provide sample implementations demonstrating common use cases.
Ensuring comprehensive documentation assists other developers in understanding and utilizing custom widgets effectively, promoting consistency across projects.
By focusing on these strategies, developers can maintain high-quality custom widgets that enhance app functionality while remaining efficient and easy to work with.
Conclusion
Creating custom widgets in Flutter opens up endless possibilities for app developers. With custom widgets, you can create user experiences that are one-of-a-kind and captivating.
While pre-built widgets are convenient, the true power of the Flutter widget catalog lies in its ability to adapt and create custom solutions. This flexibility empowers developers to customize functionality and design according to their specific requirements.
It’s important to stay updated with the latest trends and best practices in Flutter development as the framework continues to evolve. Embracing these changes will ensure that your applications remain innovative and efficient.