Detailed Guide to Understand Node.JS Memory Leaks
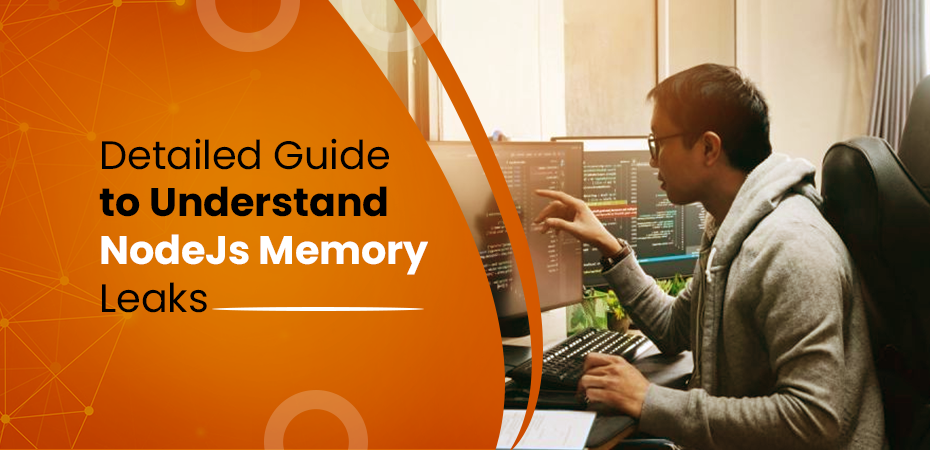
Memory management is a critical aspect of developing efficient and stable applications. In the world of Node.js, memory leaks can be a silent killer, gradually slowing down your application and eventually leading to crashes. Whether you’re a seasoned developer or just getting started, understanding how Node.js handles memory and how to detect and prevent memory leaks is crucial.
In this guide, we’ll dive deep into Node.js memory leakage, covering everything from the basics of memory management to advanced techniques for debugging and prevention. By the end of this blog, you’ll have a solid understanding of how to keep your Node.js applications running smoothly and efficiently.
Introduction to Memory Management in Node.js
Before we dive into memory leaks meaning or how to find a memory leak and how to fix a memory leak, let’s start with a brief overview of memory management in Node.js. Memory management refers to how an application handles the allocation, use, and release of memory during its execution. Proper memory management ensures that your application runs efficiently, without consuming excessive resources or crashing unexpectedly.
In Node.js, memory management is handled by the V8 JavaScript engine, which is responsible for allocating memory to your application and freeing it up when it’s no longer needed. However, even with this automated system in place, issues like memory leaks can still occur. Understanding and defining memory leaks, how computer memory leaks happen and how to check memory leaks is essential for maintaining the health of your application.
Understanding Memory Leaks in Node.js
Memory Leak Definition and Impact
Memory leaks occur when your application fails to release memory that is no longer needed, leading to Node’s high memory usage issues. This can eventually exhaust the available memory, causing your application to slow down, become unresponsive, or even crash.
In Node JS, memory leaks can be particularly troublesome because they often go unnoticed until they start causing significant performance issues. They can occur for a variety of reasons, including improper use of global variables, circular references, or even poorly managed event listeners.
The impact of memory leaks can be severe, especially in long-running applications or those that handle a large volume of requests. Over time, memory leaks can lead to increased latency, higher server costs, and a poor user experience.
Node.js Memory Management Basics
Node.js and V8 Engine Overview
At the heart of Node.js memory management is the V8 JavaScript engine, which powers both Node.js and the Chrome browser. V8 is responsible for executing your JavaScript code and managing the memory used by your application.
V8 divides memory into two main regions: Stack and Heap.
- Stack Memory: The Stack is used for storing static data such as function frames, primitive values, and pointers to objects stored in the Heap. The Stack is managed automatically by the operating system, making it a fast and efficient way to store small amounts of data.
- Heap Memory: The Heap is where dynamic data, such as objects and arrays, are stored. The Heap is managed by the V8 garbage collector, which is responsible for freeing up memory that is no longer in use.
Garbage Collection in Node.js
Garbage collection is the process by which the V8 engine automatically frees up memory that is no longer referenced by your application. This helps prevent memory leaks by ensuring that unused memory is returned to the system.
V8 uses a generational garbage collection algorithm, which divides objects in the Heap into two categories: the Young Generation and the Old Generation.
- Young Generation: New objects are allocated in the Young Generation, which has a relatively small size (1 to 8 MB). Garbage collection in the Young Generation is quick and efficient.
- Old Generation: Objects that survive garbage collection in the Young Generation are promoted to the Old Generation. The Old Generation is larger, and garbage collection here is slower and more expensive, which can impact application performance.
V8 uses two main algorithms for garbage collection:
- Scavenge Collection: This is a fast algorithm that runs on the Young Generation. It quickly collects objects that are no longer in use and frees up memory.
- Mark-Sweep Collection: This is a slower algorithm that runs on the Old Generation. It marks objects that are still in use and sweeps away those that are not.
While garbage collection is automated, it’s not foolproof. Certain coding practices can lead to memory leaks, even with garbage collection in place. Understanding how V8 manages memory is the first step in identifying and preventing these leaks.
Common Causes of Memory Leaks in Node.js
Global Variables
One of the most common causes of memory leaks in Node.js is the misuse of global variables. Global variables are variables that are accessible from anywhere in your application. While they can be convenient, they can also lead to memory leaks if not managed properly.
Global variables in Node.js are never garbage collected as long as the application is running. This means that any objects referenced by global variables, along with their child objects, remain in memory indefinitely. Over time, this can lead to significant memory usage, especially if the global variables reference large objects or data structures.
Best Practices to Avoid Global Variable Leaks:
- Minimize Use: Avoid using global variables unless absolutely necessary.
- Use Local Scope: Whenever possible, use local variables within functions or modules to limit the scope and lifespan of your variables.
- Manual Cleanup: If you must use global variables, set their values to null when they are no longer needed to allow for manual garbage collection.
Multiple Object References
Another common cause of memory leaks is having multiple references to the same object. This can occur when different parts of your application hold onto references to the same object, preventing it from being garbage collected.
For example, if an object is referenced by both a global variable and a closure, and the global variable is deleted but the closure still holds a reference, the object will not be garbage collected, leading to a memory leak.
Prevention Strategies:
- Reference Counting: Keep track of how many references exist to a particular object. If an object is no longer needed, ensure that all references to it are removed.
- Avoid Circular References: Be cautious of creating circular references, where two or more objects reference each other, as this can prevent garbage collection.
Closures
Closures are a powerful feature in JavaScript, allowing functions to retain access to variables from their containing scope. However, they can also lead to memory leaks if not managed carefully.
When a closure holds a reference to a large object in the Heap, it keeps that object in memory if the closure is in use. This can result in a memory leak if the closure is kept alive longer than necessary.
Avoiding Closure-Related Leaks:
- Limit Scope: Ensure that closures only reference the variables they need, and avoid referencing large objects unless absolutely necessary.
- Manual Cleanup: If a closure is no longer needed, remove any references to it to allow for garbage collection.
Timers and Event Handlers
Timers (such as setTimeout and setInterval) and event handlers can also cause memory leaks if they are not properly managed. If a timer or event handler references a large object, that object will remain in memory as long as the timer or event handler is active.
Best Practices:
- Clear Timers: Always clear timers using clearTimeout or clearInterval when they are no longer needed.
- Remove Event Listeners: Remove event listeners using removeEventListener when they are no longer needed to free up memory.
Detecting and Debugging Memory Leaks
Tools for Memory Leak Detection
Detecting memory leaks can be challenging, but there are several tools available that can help you identify and diagnose issues in your Node.js application.
- Memwatch: This is an older tool, but it can still be useful for detecting memory leaks. Memwatch emits leak events if it detects a consistent increase in Heap memory usage over multiple garbage collection cycles.
- Heapdump: Heapdump allows you to take snapshots of your application’s Heap memory at different points in time. You can then analyze these snapshots using tools like Chrome DevTools to identify objects that are taking up a lot of memory.
- Node Inspector: Node Inspector is a powerful tool that allows you to debug a running Node.js application. You can use it to inspect memory usage, set breakpoints, and take Heap snapshots.
Live Monitoring of Memory Usage
While tools like Heapdump are great for analyzing memory usage after the fact, live monitoring provides a more proactive approach to managing memory leaks. By monitoring your application’s memory usage in real-time, you can identify issues before they become critical.
Using Chrome DevTools:
- Chrome DevTools, which shares the V8 engine with Node.js, allows you to monitor memory usage in real-time. You can use the “Allocation instrumentation on timeline” feature to track memory allocation as your application runs.
- By recording memory usage over time, you can identify patterns and spikes that may indicate a memory leak.
Debugging Example: Step-by-Step Guide
Let’s walk through a practical example of how to debug a memory leak, which include inspecting NodeJS using Chrome DevTools.
- Start Your Node.js App: Run your app with the –inspect flag (e.g., node –inspect app.js). This enables the debugger.
- Open Chrome DevTools: In Chrome, go to chrome://inspect and click “Inspect” under your app.
- Take Heap Snapshots: In the “Memory” tab, take a heap snapshot to capture the memory usage.
- Monitor Memory Usage: Interact with your app and periodically take more heap snapshots.
- Compare Snapshots: Look for objects that keep growing between snapshots, as these could be causing the leak.
- Identify the Source: Click on the growing objects to see where they are being allocated in your code.
- Fix the Leak: Once identified, modify your code to release the memory properly.
Best Practices for Preventing Memory Leaks
Efficient Use of Stack and Heap Memory
To prevent memory leaks, it’s essential to use both Stack and Heap memory efficiently. Here are some tips to help you do that:
- Avoid Unnecessary Heap References: Whenever possible, avoid referencing Heap objects from the Stack. This will reduce the likelihood of memory leaks.
- Destructure Objects: Instead of passing entire objects to functions, destructure them and pass only the fields you need. This reduces the memory footprint and makes your code more efficient.
- Short-Lived Variables: Keep variables short-lived and limit their scope. This ensures that they are garbage collected promptly.
Proper Use of Closures, Timers, and Event Handlers
Closures, timers, and event handlers are common sources of memory leaks, but with careful management, you can avoid issues.
- Use Closures Sparingly: Only use closures when necessary and ensure they do not reference large objects unless absolutely required.
- Manage Timers: Clear timers when they are no longer needed and avoid keeping them active indefinitely.
- Clean Up Event Handlers: Always remove event listeners once they have completed their task to prevent memory leaks.
Conclusion
In this guide, we’ve explored the ins and outs of Node JavaScript memory leaks, from understanding the basics of memory management to detecting, debugging, and preventing leaks. By following the best practices outlined in this guide, you can significantly reduce the risk of memory leaks in your Node.js applications, ensuring they run smoothly and efficiently.
Memory leaks can be tricky to identify and resolve, but with the right tools and knowledge, you can keep your applications healthy and performant. Whether you’re using tools like Chrome DevTools, or other debugging utilities, staying vigilant about memory usage will help you build more reliable and scalable applications.