Everything You Need To Know About Node JS 22
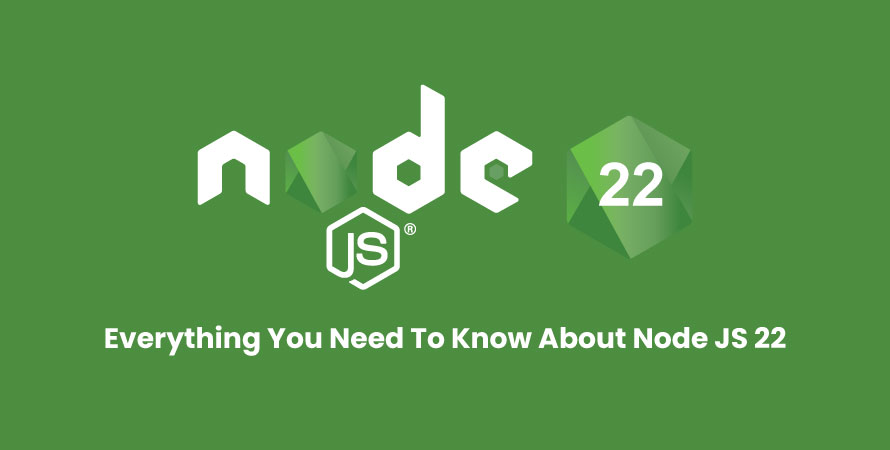
Node.js version 22 is a major update to the popular JavaScript runtime, built on Chrome’s V8 engine. Nodejs was released in April 2024, this version brings several new features and improvements aimed at enhancing performance and developer experience.
The significance of the latest version of Node JS 22 in the developer community cannot be overstated, as it introduces tools and functionalities that streamline development workflows and boost application performance.
In this article, you will learn about:
- Key Features of Node.js 22: An in-depth look at the latest enhancements such as ECMAScript Modules (ESM) support, nodejs WebSocket client, updated V8 JavaScript engine, Maglev compiler, and more.
- Benefits for Developers: How these new features positively impact developers in terms of performance, ease of use, and overall productivity.
- Transitioning to Node.js 22: Considerations for upgrading from previous versions and understanding end-of-life timelines.
By diving into these topics, you’ll gain a comprehensive understanding of what Node.js 22 offers and how it can improve your development processes.
Key Features of Node.js 22
ECMAScript Modules (ESM) Support
Node js releases significant enhancements to ECMAScript Modules (ESM) support, making it easier for developers to transition from CommonJS to ESM. Previously, importing ESM modules required asynchronous import() calls, which could lead to complexities in synchronous codebases. Node 22 now allows requiring ESM graphs using the traditional require() function.
Detailed Explanation of ESM Support in Node.js 22
- Synchronous Import Capability: Developers can now use require() to synchronously import ESM modules. This change simplifies integrating ESM into existing codebases that rely heavily on synchronous imports.
- Unified Module System: With improved ESM support, Node.js 22 bridges the gap between CommonJS and ES modules. It offers a more consistent and streamlined module system that aligns with modern JavaScript standards.
Benefits for Developers Transitioning to ESM
- Simplified Migration: The ability to use require() with ESM reduces friction when migrating large codebases from CommonJS to ESM. Developers can incrementally adopt ESM without needing to refactor entire sections of their applications.
- Enhanced Compatibility: Better ESM support ensures greater compatibility with front-end frameworks and libraries that already use ES modules. This facilitates seamless integration across the stack.
- Performance Improvements: Native support for ESM in Node.js 22 can lead to performance gains by leveraging optimized module loading mechanisms inherent in the V8 engine.
Native WebSocket Client
Node.js 22 introduces a native WebSocket client, eliminating the need for external dependencies to manage real-time communication. These Node js and websockets are built directly into the core, allowing developers to create and manage WebSocket connections seamlessly.
Advantages for Developers Building Real-Time Applications
- Reduced Dependency Management: With a native solution, there’s no need to rely on third-party libraries. The websocket in Node JS simplifies the development environment and reduces potential security vulnerabilities.
- Performance Enhancements: Leveraging a built-in WebSocket client results in faster execution and lower overhead compared to using external modules.
- Ease of Use: The integrated WebSocket Nodejs client offers a consistent API that aligns with other Node.js components, streamlining the development process for real-time applications like chat apps, gaming servers, and live data feeds.
javascript const ws = new WebSocket(‘ws://example.com/socket’);
ws.on(‘open’, function open() { console.log(‘connected’); ws.send(Date.now()); });
ws.on(‘message’, function incoming(data) { console.log(data); });
The inclusion of Node websockets in Node.js 22 reflects a significant step towards enhancing the ecosystem’s support for modern web applications.
Updated V8 JavaScript Engine
Nodejs latest version brings significant enhancements to the V8 JavaScript engine, now updated to version 12.4. This update introduces several key features that improve performance and add new capabilities:
- WebAssembly Garbage Collection: Enhances memory management for WebAssembly applications, making them more efficient.
- New Array Methods: Methods like Array.fromAsync facilitate asynchronous operations with arrays, streamlining code and improving readability.
- Iterator Helpers: Simplifies iterator operations with new helper functions, reducing boilerplate code.
These updates to the V8 engine elevate the performance of Node.js applications, enabling developers to write more efficient and maintainable code. Enhanced memory management and new array methods contribute to a smoother and more responsive user experience. The iterator helpers simplify complex iterator tasks, allowing for more straightforward code implementation.
Maglev Compiler
The Maglev compiler is a standout feature in Node.js 22, aimed at enhancing the performance of short-lived CLI applications. This new Just-In-Time (JIT) compiler is enabled by default on supported architectures.
Key Functionalities:
- Optimized Performance: The Maglev compiler improves execution speed by optimizing code paths that are frequently executed but do not benefit significantly from long-term optimizations.
- Low Overhead: Designed to have minimal startup overhead, making it ideal for CLI tools and scripts that execute quickly and terminate.
Impact on CLI Applications:
- Faster Execution Times: Developers will notice a reduction in execution times for command-line utilities and scripts, leading to more efficient development workflows.
- Enhanced Responsiveness: Applications benefit from quicker response times, which is particularly beneficial for tasks that require immediate feedback.
The introduction of the Maglev compiler adds to the array of key features in Node.js 22, improving both performance and usability.
Package.json Script Execution Enhancements
Executing scripts directly from package.json in Node.js watch 22 streamlines development workflows significantly. Developers can use the command node –run <script-in-package-json> to execute scripts defined in the package json scripts section of their package.json file. This simplifies script management by:
- Reducing overhead: No need to install additional tools like npm or yarn just to run scripts.
- Improving consistency: Ensures that the execution environment of the package json engines is consistent across different setups.
- Enhancing simplicity: Provides a more straightforward way to manage and run development tasks.
Key features of the latest version of Node.js 22, such as ESM support, WebSocket client, and V8 engine updates, complement these enhancements, making it a robust choice for developers focused on performance and usability.
Stable Watch Mode
Node.js 22 introduces a stable watch mode feature that greatly enhances the development workflow. This mode automatically restarts applications when watched files are modified, making it much easier to see changes in real-time without manual restarts.
Benefits for Developers:
- Improved Productivity: Developers save time as they no longer need to stop and start their applications manually after every change.
- Error Reduction: Immediate feedback on code changes helps catch errors early in the development process.
- Ease of Use: The stable watch mode is simple to implement, requiring minimal configuration.
javascript // Example usage: node –watch app.js
This command starts your application in watch mode, monitoring changes to app.js and any dependencies. This seamless integration into the Node.js environment makes developing and debugging more efficient and less error-prone.
Performance Improvements
Node.js 22 brings significant performance improvements, especially with the increased default High Water Mark for streams. This value has been raised from 16KiB to 64KiB.
Benefits:
- Enhanced Streaming Performance: The larger buffer size means that more data can be read or written in a single operation, leading to faster streaming.
- Optimized Throughput: Higher throughput is achieved due to fewer interruptions for refilling the buffer.
Trade-offs:
- Increased Memory Usage: While performance is boosted, the trade-off comes in the form of higher memory consumption. This might impact applications with limited resources or those running on constrained environments.
javascript const fs = require(‘fs’); const readableStream = fs.createReadStream(‘largefile.txt’, { highWaterMark: 64 * 1024 }); // 64KiB buffer
This adjustment aligns with other improvements like ESM support and V8 engine updates, reflecting Node.js’s aim to balance performance and usability efficiently.
New Glob Functions
Node.js 22 introduces new glob Nodejs and globSync functions within the node:fs module, enhancing file path matching capabilities. These functions enable developers to use pattern matching to identify files and folders based on specified criteria, streamlining file operations.
Utility in File Operations
- glob(pattern, [options], callback): Asynchronously matches file paths based on the provided pattern. This function is highly useful for applications requiring dynamic file management.
- globSync(pattern, [options]): Synchronously performs pattern matching, ideal for scenarios where immediate results are necessary, such as during script execution or configuration loading.
By integrating these functions directly into Node.js 22, developers gain a powerful tool for efficient file handling without relying on external libraries. This enhancement contributes significantly to the key features of Node.js 22, offering improved performance and usability.
AbortSignal Enhancements
Node.js 22 introduces significant improvements to AbortSignal creation, a key feature impacting performance, especially in fetch operations. These enhancements provide Node js developers with more efficient ways to handle abortable tasks.
1. Optimized Creation
The process of creating AbortSignal instances is now more streamlined. This optimization results in reduced overhead, which is particularly beneficial in high-performance and real-time applications.
2. Improved Fetch Operations
With the enhanced AbortSignal, fetch operations become more responsive and can be aborted more swiftly, leading to better resource management and quicker cleanup of unused processes.
3. Enhanced Test Runners
The improvements also extend to test runners that rely on AbortSignal. Faster abort signal handling leads to quicker test execution and improved overall testing efficiency.
The advancements in AbortSignal creation are part of the broader efforts in Node.js 22 to boost performance and usability, adding another layer of robustness to the JavaScript runtime environment.
Transitioning to Node.js 22
Understanding End-of-Life Timelines
When upgrading to Node.js 22, it’s important to know the end-of-life (EOL) timelines for previous versions. Here’s how Node.js manages its releases:
- Current Release Phase: Node.js 22 is in the Current release phase until October 2024.
- Long-Term Support (LTS): After October 2024, it moves into LTS, providing stability and extended support until April 2027.
Key Considerations for Your Transition Plan
Your transition plan should take into account the following:
1. Node.js 18 EOL
Node.js 18 will reach its end-of-life in April 2025. If your applications are currently running on this version, you should start testing their compatibility with Node.js 22.
2. Testing and Compatibility
Start testing your applications with Node.js 22 as soon as possible. This will help you identify and fix any breaking changes or deprecated features in your code.
3. Security and Maintenance
Upgrading to Node.js 22 ensures that you continue to receive security patches and critical updates. Older versions may not get these important updates after they reach EOL.
4. Tooling and Dependencies
Make sure all your dependencies work with Node.js 22. You may need to update your build tools, libraries, and packages as well.
Why Upgrading Matters?
Upgrading to Node.js 22 isn’t just about keeping up with the latest version; it’s also about taking advantage of new features, better performance, and ongoing support. A well-planned transition will ensure a smooth upgrade process with minimal disruptions.
Conclusion
Node.js 22 marks a significant milestone in the evolution of Node.js development, especially with the introduction of ECMAScript Modules (ESM) support. This addition modernizes JavaScript development by allowing developers to leverage the same module system used in modern browsers, making it easier to build cross-platform applications. Alongside ESM, the inclusion of a native WebSocket client further strengthens Node.js for real-time applications, while the upgraded V8 engine enhances performance and compatibility with the latest JavaScript features.
At BuildOffshore team, these advancements are particularly exciting for Node.js development. Key features in Node.js 22 such as the Maglev Compiler, which boosts performance for short-lived CLI applications, and the stable Watch Mode, which simplifies development workflows, are game-changers. Performance enhancements like the increased High Water Mark for streams, improved glob functions, and AbortSignal improvements for faster fetch operations make this version more efficient and robust. These updates empower BuildOffshore developers to build high-performance, scalable applications with greater ease and flexibility.