How To Create Dynamic Forms Using Angular JS
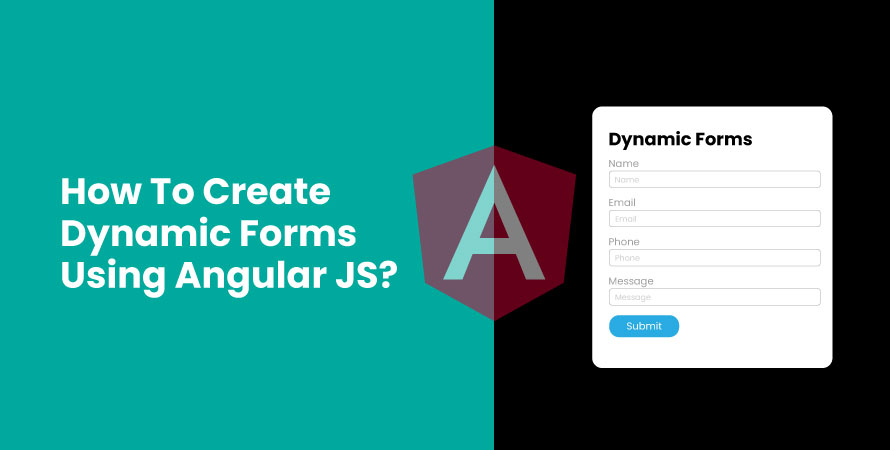
Creating dynamic forms in web development allows for flexible and interactive user interfaces where form fields can be added, removed, or modified based on user input or other conditions. This adaptability is crucial for applications that require user-specific input forms, such as surveys, registration forms, and many types of data entry systems.
AngularJS stands out as a robust framework for building these dynamic forms. Its powerful two-way data binding, directives like ng-repeat and ng-show, and ease of integrating controller functions make it an excellent choice for managing complex form behaviors. Using AngularJS simplifies updating the UI in response to changes in the underlying data model, ensuring that your forms remain synchronized with the application’s state.
This blog will guide you through:
- Setting Up the Project: Instructions for creating your project folder and setting up the index.html file.
- Creating Dynamic Form Fields: Using the ng-repeat directive to generate form controls dynamically.
- Implementing Controller Functions: Managing dynamic entries with functions like addName() and removeName(index).
- Conditional Display: Utilizing the ng-show directive for conditional display of form sections.
- Form Submission and Validation: Capturing and processing entered data while ensuring validation.
- Styling and Responsiveness: Applying CSS techniques to enhance visual appeal and ensure device compatibility.
- Reactive Forms (Optional): Transitioning to reactive forms in Angular formcontrol for more advanced dynamic form management.
By the end of this blog, you’ll have a comprehensive understanding of creating dynamic forms using Angular form builder, equipped with practical examples and best practices.
Setting Up the Project
Creating a New Project Folder
Begin by setting up a dedicated project folder for your AngularJS application. This will help keep all related files organized. Follow these steps:
- Create a New Directory: bash mkdir dynamic-forms-angularjs cd dynamic-forms-angularjs
- Initialize the Project: Create an index.html file within this directory to serve as the entry point for your application.
Setting Up index.html
Your index.html file will include necessary AngularJS libraries and provide the basic structure for your application:
- Basic HTML Structure: html
- Including AngularJS: Add the AngularJS library to your project by including it in your <head> section through a CDN link, as shown above.
- Basic AngularJS Setup: Define your AngularJS application module in a separate JavaScript file (e.g., app.js).
Initialize the application and define a controller to manage form logic.
Example app.js:
var app = angular.module('dynamicFormsApp', []); app.controller('FormController', function($scope) { $scope.names = []; $scope.addName = function() { $scope.names.push({ name: '', gender: '', interests: [] }); }; $scope.removeName = function(index) { $scope.names.splice(index, 1); }; });
By setting up your project folder and configuring index.html, you establish a solid foundation for building dynamic Angular form control. This preparation ensures that you can seamlessly integrate form functionalities as you proceed with development.
Creating Dynamic Form Fields with ng-repeat Directive
Dynamic forms in AngularJS provide the flexibility to render form controls based on data models. The ng-repeat directive is a powerful tool for achieving this by iterating over an array of objects and generating form elements dynamically.
Utilizing the ng-repeat Directive
The ng-repeat directive can loop through a list of items, creating a new instance of the specified HTML element for each item. This is particularly useful for generating multiple input fields, radio buttons, or checkboxes without manually coding each one.
html
In this example:
An AngularJS application module named dynamicFormApp is created.
A controller named dynamicFormController is linked to manage the dynamic form behavior.
An angular 14 add items to arraylist example accessing various fields.
Example Model Including Fields like Name, Gender, Interests
A typical model might include personal details such as name, gender, and interests. Here’s how you can define such a model in your controller:
javascript
var app = angular.module('dynamicFormApp', []); app.controller('dynamicFormController', function($scope) { $scope.formFields = [ { label: 'Name', type: 'text', value: '' }, { label: 'Gender', type: 'radio', value: '', options: ['Male', 'Female'] }, { label: 'Interests', type: 'checkbox', value: [], options: ['Sports', 'Movies', 'Travel'] } ]; });
This model features:
A text input for “Name”.
Radio buttons for “Gender”.
Checkboxes for “Interests”.
Binding Data to Input Elements using AngularJS Data Binding
AngularJS allows seamless data binding between the model and view. When using the ng-model directive, any changes in the input fields automatically update the respective model properties.
To handle different types of inputs like text, radio buttons, and checkboxes, you can extend your form as follows:
html
<!– Text Input –>
<input ng-if=”field.type === ‘text'” type=”text” ng-model=”field.value” />
<!– Radio Buttons –>
<div ng-if=”field.type === ‘radio'”>
<label ng-repeat=”option in field.options”>
<input type=”radio” ng-model=”field.value” value=”{{option}}”> {{option}}
</label>
</div>
<!– Checkboxes –>
<div ng-if=”field.type === ‘checkbox'”>
<label ng-repeat=”option in field.options”>
<input type=”checkbox” ng-model=”selectedInterests[option]” /> {{option}}
</label>
</div>
With this setup:
Text inputs are rendered directly.
Radio buttons are generated based on the options provided.
Checkboxes are created similarly with their options.
AngularJS’s two-way data binding ensures that any updates to these input elements reflect immediately in the controller’s scope.
This approach provides a robust foundation for creating Angular dynamic forms that adapt easily to various requirements by simply modifying the underlying data model.
Implementing Controller Functions for Dynamic Behavior in Forms
Managing dynamic form builder entries in a form requires effective controller functions that handle the addition and removal of form fields. AngularJS offers a straightforward way to implement these functions within a controller.
Implementing Add and Remove Functions
Controller functions are essential for managing dynamic behavior in forms. To add new entries dynamically, you can create an addName() function. This function will push a new entry into an array, such as an array of names.
javascript
app.controller('FormController', function($scope) { $scope.names = [{ name: '' }]; $scope.addName = function() { $scope.names.push({ name: '' }); }; $scope.removeName = function(index) { $scope.names.splice(index, 1); }; });
In this example:
addName(): Pushes a new object { name: ” } into the $scope.names array.
removeName(index): Removes the element at the specified index from the $scope.names array.
Connecting Controller Logic with the View
To see real-time updates in the view, bind these controller functions to buttons within your HTML. Use Angular forms formgroup directives to ensure proper data binding between your model and view.
html
In this snippet:
Each input field is dynamically generated using ng-repeat, iterating over $scope.names.
- The Remove button invokes removeName($index) to delete the specific entry.
- The Add Name button calls addName() to append a new entry.
Conditional Display Using ng-show Directive in AngularJS Forms
The ng-show directive in AngularJS is a powerful tool for creating dynamic and interactive forms by conditionally rendering sections based on user input or selection. This enhances the user experience by only displaying relevant information at the right time.
How to Use ng-show?
To utilize the ng-show directive, you need a boolean expression that determines whether an element should be displayed. When the expression evaluates to true, the element is shown; otherwise, it is hidden.
Example
An Angular form example describes imagining we have a formcontrolname in Angular material where users can specify their interests. Based on their selection, different sections of the form in angular material will appear.
html
In this example:
We have two checkboxes that bind to the interests model.
The ng-show directive is used to conditionally render sections based on the state of these checkboxes.
If a user selects “Sports”, the sports-related fields are displayed. Similarly, selecting “Movies” shows the movie-related fields.
Benefits of Using ng-show
- Improves User Experience: By only showing relevant sections, you reduce clutter and make forms easier to navigate.
- Efficient Rendering: Elements controlled by ng-show are still part of the DOM but hidden from view, which can be more efficient than completely adding/removing elements.
Implementing conditional display with ng-show makes your Angular forms array smarter and more responsive to user interactions. This formarray Angular method can be expanded further for more complex scenarios involving multiple conditions and nested elements.
Handling Form Submission and Validation in AngularJS Forms
Effective form submission handling and form validation are crucial aspects of creating dynamic forms in AngularJS. This involves capturing user-entered data, processing it, and ensuring the form meets certain criteria before submission.
Capturing Entered Data on Form Submission
To capture data upon form submission:
- Define a Controller Function: Create a function within your AngularJS controller that will handle the form submission. javascript app.controller(‘FormController’, function($scope) { $scope.formData = {};
- Bind the Function to the Form: Use the ng-submit directive to bind this function to your form element. html
When the user submits the Angular form submit, submitForm is invoked, logging and processing the formData.
Processing and Resetting Form State
After processing the captured data, you might want to reset the form control angular state:
- Resetting Form Fields: Clear out the formData object. javascript $scope.submitForm = function() { console.log($scope.formData);
// Resetting form data
$scope.formData = {};
// Optionally reset form validation states
$scope.myForm.$setPristine();
$scope.myForm.$setUntouched(); }; - HTML Binding for Reset: Ensure your HTML reflects these changes. HTML
Validating User Inputs Before Final Submission
Validation ensures users provide correct and complete information:
- Built-in Validation Directives: Leverage AngularJS’s built-in directives like required, minlength, and maxlength. html
- Custom Validation Logic: Implement custom validation logic within your controller. javascript app.controller(‘FormController’, function($scope) { $scope.submitForm = function() { if ($scope.myForm.$valid) { console.log(“Valid:”, $scope.formData); // Proceed with submission logic here } else { alert(“Invalid input”); } }; });
- Conditional Classes for Feedback: Apply conditional classes for visual feedback based on validation states. html
Efficiently handling data capture and validation not only enhances user experience but also ensures data integrity within dynamic forms created using Angular formarray.
Styling and Making AngularJS Forms Responsive Across Devices
Importance of CSS for Enhancing the Visual Appeal of Dynamic Forms
CSS styling in forms plays a crucial role in creating a user-friendly interface. By applying well-thought-out styles, you can:
- Improve readability: Use font sizes, colors, and spacing that ensure the form is easy to read.
- Highlight important elements: Draw attention to required fields or critical information using color coding and borders.
- Maintain visual consistency: Ensure your form aligns with the overall design language of your application for a cohesive look.
Techniques for Ensuring Responsiveness Across Different Devices and Screen Sizes
Ensuring responsive design involves making your formcontrol Angular array adaptable to various screen sizes. Some effective techniques include:
1. Media Queries
css @media (max-width: 600px) { .form-container { flex-direction: column; } }
This example changes the layout to a single column on smaller screens.
2. Fluid Grid Layouts
css .form-container { display: flex; flex-wrap: wrap; }
.form-field { flex: 1 1 100%; margin-bottom: 10px; }
@media (min-width: 768px) { .form-field { flex: 1; } }
This setup ensures that form fields stack vertically on small screens and align in rows on larger screens.
3. Viewport Meta Tag
html
Including this tag ensures that your form scales appropriately across different devices.
4. Flexible Units
Utilize relative units like percentages, em, or rem instead of fixed units (px) to allow components to scale naturally with screen size changes.
5. Responsive Input Elements
css input[type=”text”], select { width: calc(100% – 20px); padding: 10px; margin-bottom: 10px; }
@media (min-width: 768px) { input[type=”text”], select { width: calc(50% – 20px); } }
These styles adjust the width of the input elements based on screen size, ensuring they remain usable without horizontal scrolling.
By integrating these CSS techniques, dynamic forms created using Angular JSonpath will not only be visually appealing but also provide a seamless user experience across all devices.
Conclusion
Creating dynamic forms with AngularJS or transitioning to reactive forms in Angular provides a flexible, robust solution for modern web applications. AngularJS offers seamless data binding, with directives like ng-repeat and ng-show to dynamically generate and control form fields. Angular’s reactive forms, with FormGroup and other features, emphasize scalability and ease of validation. Mastering both approaches enhances your ability to build sophisticated, responsive applications. Whether you’re optimizing existing forms or developing new ones, these skills are essential.
Need expert help? Partner with Build Offshore Team to accelerate your project with top-tier development talent.