Why Should You Use Vue.js for Front-End Development?
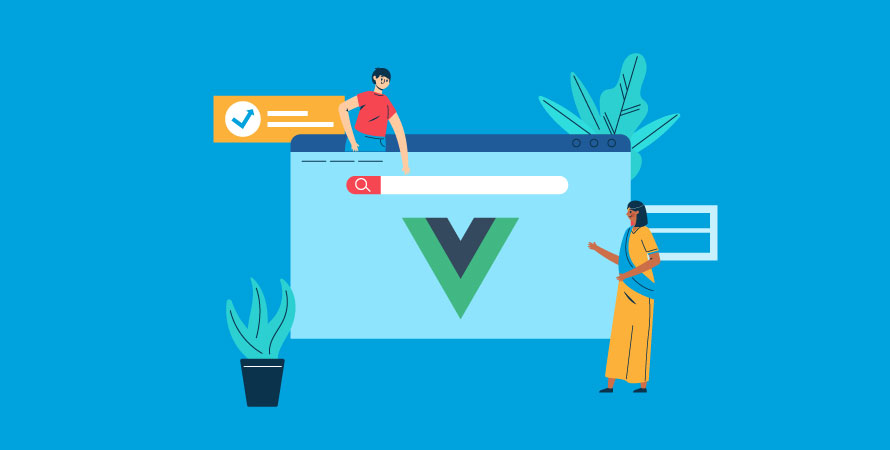
Vue.js is a progressive JavaScript framework used for building user interfaces. Vue framework has gained significant popularity in the frontend development community due to its simplicity and flexibility. Unlike other monolithic frameworks, Vue JS is designed to be incrementally adoptable, meaning you can use as much or as little of it as you need.
Here, in this blog, you will know whether is Vue still relevant in the frontend development process or not. If you are a front-end developer, then it is crucial to learn about vue javascript framework in detail.
Why Use a Frontend JavaScript Framework?
Using a frontend Vue JS framework like Vue.js greatly enhances development productivity and maintainability. These Vuejs frameworks can be easily integrated and provide:
- Component-based architecture: Encourages code reusability and modularity.
- Declarative rendering: Simplifies the process of updating the DOM element.
- State management: Helps manage the state of your application in real-time more efficiently.
Reactivity in Vue.js
One of the standout features of Vue.js is its reactivity system. Reactivity enables seamless updates of the user interface when underlying data changes. This is achieved through:
- Reactive data binding: Automatically synchronizes data changes between the model and the view.
- Computed properties: Automatically recalculates values based on reactive dependencies.
- Watchers: Observe changes in data and perform side effects accordingly.
These features make Vue.js an excellent choice for building dynamic, responsive user interfaces.
Advantages of Using Vue.js for Frontend Development
Rich Ecosystem and Easy Learning Curve
The front-end Vue js developers have a wide range of tools and libraries that make development easier. It’s beginner-friendly, but also powerful enough for experienced Vuejs developers.
Efficient Development with Webpack
Vue.js works well with Webpack, a tool that bundles modules together. This means faster development with features like automatic code updates and splitting code into smaller files.
Component-Based Architecture and Reusability with Single-file Components
Vue software uses a component-based structure, which means you can reuse code across your project. Single-file components (SFC) make it even easier by combining the HTML, CSS, and JavaScript for each component into one file.
Maintainable Styling with Scoped CSS
In Vue js documentation, you can write CSS styles that only apply to specific components. This prevents styles from affecting other parts of your app and makes it easier to manage your styling.
Flexible State Management with Vuex
For managing data in your app, Vue.js has Vuex. It’s like a central storage space where all your components can access and update information. This makes it easier to keep track of your app’s state and debug any issues.
Benefits of Performance Optimization Techniques in Vue.js
Vue frontend development includes features that help improve how fast your app loads and how smoothly it runs:
- Lazy-loading: Only loading parts of your app when they’re needed, instead of everything at once.
- Asynchronous components: Loading components in the background while the main content is being displayed.
- Efficient reactivity systems: Updating only the necessary parts of your app when data changes, instead of refreshing the whole page.
These optimizations result in a better user experience overall.
Improving SEO with Server-side Rendering (SSR) and Nuxt.js
When using Vue.js for server-side rendering (SSR), your web pages are generated on the server before being sent to the client. This can greatly improve SEO by making it easier for search engines to crawl and index your content.
Nuxt.js, a framework built on top of Javascript Vue, makes implementing SSR easier and also offers features like static site generation for even better SEO performance.
By taking advantage of these benefits, developers can build high-performance, easy-to-maintain, and scalable applications using Vue.js.
Exploring Key Features of Vue.js
Managing Data Flow with Props
In Vue.js, managing data flow effectively is essential for building maintainable applications. This is where props come into play. Props are custom attributes that you can register on a component, allowing you to pass data from a parent component down to its children. This creates a clear and structured way to manage data flow within your application.
Here’s an example of how props work:
vue
In the child component, you would define props like this:
vue
This approach makes it easier to track where data is coming from and how it flows through your application, enhancing both readability and maintainability.
Server-Side Rendering (SSR) and Nuxt.js
Vue.js supports Server-Side Rendering (SSR), a technique that improves SEO and initial load performance by rendering the application on the server before sending it to the client. SSR can be complex, but Vue’s infinite ecosystem includes Nuxt.js, a framework specifically designed to simplify the process of building server-rendered Vue applications.
Nuxt.js provides out-of-the-box support for features like:
- Automatic code splitting
- Powerful routing system
- Static site generation
With these tools, developers can optimize their applications for better performance and SEO without getting bogged down in configuration details.
Vue.js 3 Enhancements
Vue.js 3 introduces several enhancements that improve developer experience and application performance:
- Composition API: Offers a more flexible way to organize component logic.
- Improved TypeScript Support: Makes it easier to build large-scale applications with type safety.
- Smaller Bundle Size: Helps in faster load times and improved application performance.
These features make Vue.js 3 a powerful tool for modern front-end development, ensuring both efficiency and flexibility.
Comparing Vue.js with Other Major Frontend Frameworks
Understanding Different Design Philosophies: Reactivity vs. Virtual DOM
When comparing Vue.js with other major frontend frameworks like React and Angular, one of the primary distinctions lies in their design philosophies.
Vue.js: Embracing Reactivity
Vue.js embraces a reactivity system that automatically tracks changes in an application’s state and updates the DOM accordingly. This reactivity model simplifies state management and makes it easier to develop dynamic user interfaces.
React: Leveraging the Virtual DOM
React utilizes a virtual DOM to manage and optimize updates. The virtual DOM is a lightweight copy of the actual DOM, allowing React to efficiently determine the minimal number of changes needed to update the UI. This approach can be highly performant but may require more boilerplate code for state management.
Angular: Combining Concepts
Angular combines concepts from both reactivity and virtual DOM. Angular’s change detection mechanism leverages zones and observables to track state changes, while its templating syntax allows for declarative data binding similar to Vue usage.
Key Differences
When considering which framework to use, it’s important to understand the key differences:
Learning Curve
- Vue.js: Easier for beginners due to its simple API and clear documentation.
- React: Requires understanding of JSX, a syntax extension that allows mixing HTML with JavaScript.
- Angular: Steeper learning curve because of its extensive use of TypeScript and comprehensive feature set.
Community & Ecosystem
- Vue.js: Growing rapidly with a rich ecosystem of tools and libraries.
- React: Backed by Facebook, boasting a massive community and numerous third-party libraries.
- Angular: Supported by Google, known for its robust framework suitable for large-scale applications.
Understanding these differences helps developers choose the right tool based on their project requirements and familiarity with each framework’s design philosophy.
Advanced Tips and Tricks for Vue.js Development
Writing Performant Vue.js Applications with Efficient Event Handling
Efficient event handling is crucial for building performant Vue.js applications. By optimizing how events are managed within your components, you can significantly reduce unnecessary re-renders and improve overall application responsiveness.
Debouncing and Throttling
Debouncing ensures that a function is called only once after a specified delay. This is particularly useful for input fields where you don’t want the function to execute on every keystroke.
Throttling limits the execution of a function to once in a specified time period. It’s ideal for events like scrolling or resizing.
Here’s an example of how you can implement debouncing in a Vue.js component:
javascript <input @input=”debouncedInput” />
Lazy Loading / Async Components
In large applications, loading all components at once can affect performance. Vue.js supports lazy loading and asynchronous components to mitigate this issue.
Lazy Loading Routes
Lazy loading routes allows you to load components only when they are needed. Here’s an example of how you can lazy load a route in Vue.js:
javascript const routes = [ { path: ‘/about’, component: () => import(‘./components/About.vue’) } ];
This approach ensures that the About component is only loaded when the user navigates to the /about route.
Async Components
Async components are another way to lazily load parts of your application. Here’s an example of how you can create an async component in Vue.js:
javascript Vue.component(‘async-example’, function (resolve) { setTimeout(function () { resolve({ template: ‘I am async!’ }); }, 1000); });
Using async components allows you to load parts of your application on demand, improving initial load times and user experience.
These techniques not only enhance performance but also contribute to a smoother and more responsive application.
Conclusion
Vue.js is an excellent choice for front-end development because it’s easy to use, flexible, and packed with powerful features. The reactivity system in Vue.js makes it simple to create dynamic user interfaces, and its extensive ecosystem caters to various development requirements—from basic improvements to complex Single Page Applications (SPAs).
Key reasons to consider Vue.js:
- Rich Ecosystem: Tools like Vue CLI, Vuex for state management, and Nuxt.js for server-side rendering enhance productivity.
- Performance Optimization: Built-in techniques ensure fast rendering and efficient updates.
- Easy Learning Curve: Designed with simplicity in mind, making it accessible for beginners but robust enough for experienced developers.
The introduction of Vue.js 3 brings exciting features and benefits, including the Composition API for better code organization and improved TypeScript support. This makes it even more attractive for modern JavaScript projects.
By joining the Vue.js community, you not only gain access to a wealth of resources and support but also have the opportunity to contribute to one of the liveliest ecosystems in web development today.